- Angular 36
- Laravel 18
- PHP 12
- Featured 9
- Ionic 7
- Javascript 7
- Jekyll 4
- Blogging 3
- Typescript 3
- *ngFor 2
- Angular CLI 2
- Cypress 2
- Mapbox 2
- Netlify 2
- PWA 2
- RxJS 2
- ScullyIO 2
- Unit Testing 2
- AGM 1
- API 1
- API Redirect 1
- Automation 1
- Bootstrap 4 1
- CAMTEL 1
- CSRF 1
- Composer 1
- ControlValueAccessor 1
- Custom Form Control 1
- DTOs 1
- Databases 1
- Date and Time Formatting 1
- DateInterval 1
- Deep Cloning Objects 1
- Dependency Injection 1
- Directions Renderer 1
- Directions Service 1
- Directive 1
- Directives 1
- Exception Handling 1
- Exceptions 1
- Form Arrays 1
- Fragment Navigation 1
- Google Maps 1
- Google Places Autocomplete 1
- HttpErrorResponse 1
- Injection Token 1
- Javascript Date Object 1
- KaiOS 1
- Laravel Horizon 1
- Laravel Validation Errors 1
- Livewire 1
- MySQL 1
- NPM 1
- NbDialogService 1
- Nebular 1
- Nebular UI Kit 1
- Netlify CMS 1
- PDF Generation with Angular 1
- Password Reset 1
- Proxy Redirect 1
- Reactive Forms 1
- Renderer2 1
- RendererFactory2 1
- Retry Failed Requests 1
- Rouge 1
- Router Resolver 1
- RxJs 1
- SCSS 1
- Spread Operator 1
- State Management 1
- Strongly Typed Reactive Forms 1
- Testing 1
- Text Processing 1
- Url Protocol 1
- Waypoints 1
- Window Object 1
- YAML 1
- agGrid 1
- agGrid Checkbox Selection 1
- distinctUntilChanged 1
- html time 1
- jsPDF 1
- nb-sidebar 1
- regex 1
- shareReplay 1
- trackBy 1
- try/catch 1
Angular
Netlify build command for an Ionic Angular app
In the Build command
input field, enter ng build --prod
, and in the Publish directory
input field, enter www
.
Angular Service Worker: Prefetch all assets on first run
I’ve been working on the PWA version of a mobile app and it was brought to my attention that when using the PWA offline, some icons that were placed in the /src/assets
directory fail to load.
Angular: Conditionally Rendering Mapbox Markers
Say we’re using Mapbox to render a map that contains a couple of icons representing places. When the map loads for the first time, no marker should be rendered.
Ionic 4 Swipe Navigation Between Pages
Imagine in your app there’s a page with a list of items, and clicking on an item navigates to and item details page. On the details page, you’d like to be able to swipe left to bring up the previous item, or right to bring up the next item. This post aims to provide an implementation for such functionality.
Add your Proxy Configuration File to angular.json - and forget it ever existed
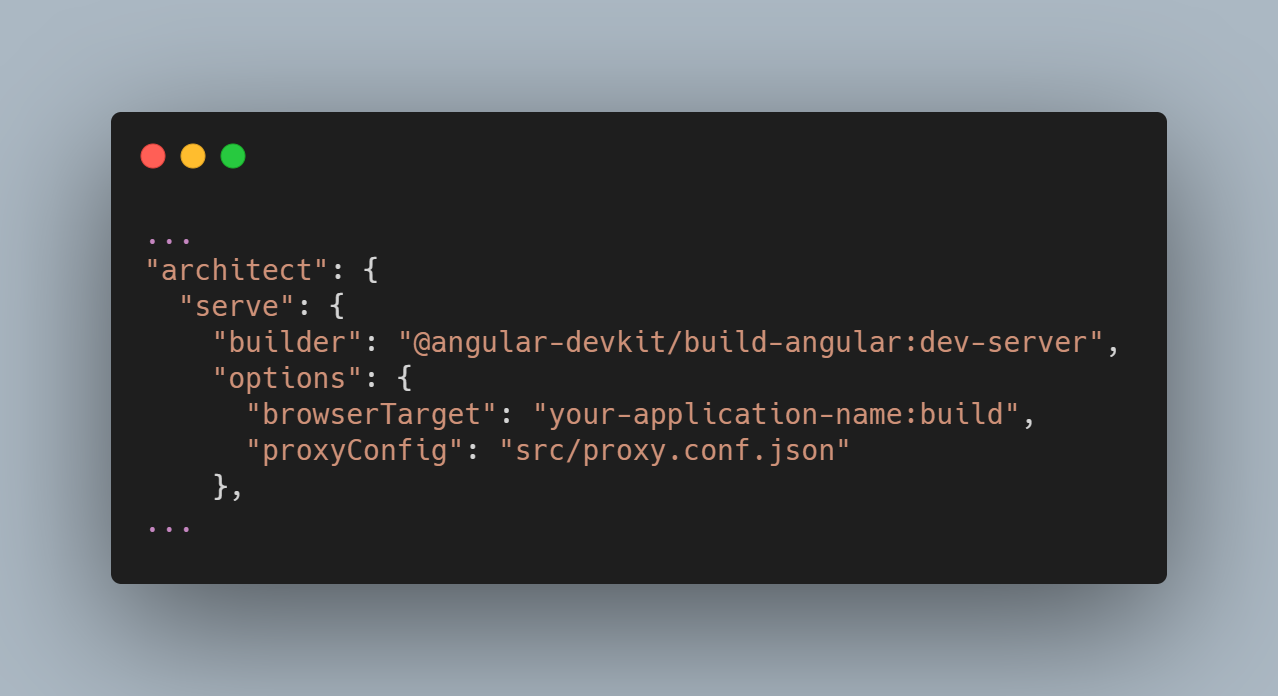
What if you never have to write the –proxy-config CLI option again when serving your Angular application?
Creating a Delayed Input Directive in Angular
Imagine in your app there’s a search input that triggers an http request on each keystroke as a user types in their query. As your userbase grows, search operations quickly become expensive due to the increased traffic to your server.
Creating a Lazy Loaded Image Cropping Modal Component with Ionic 5 and ngx-image-cropper
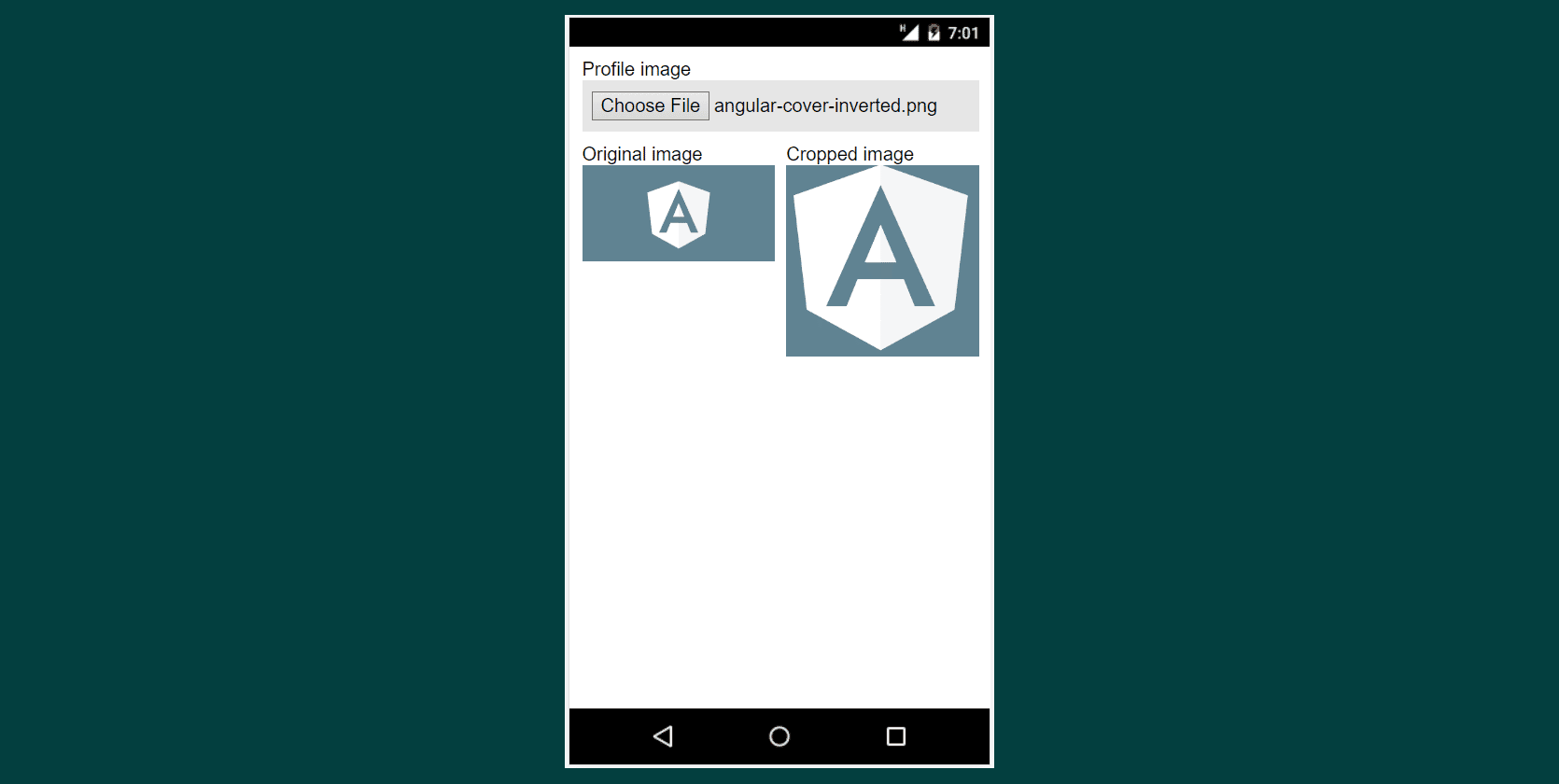
Demo and source code
Debugging ScullyIO Rendering and Network Errors with the help of puppeteerLaunchOptions
With my Angular app’s Scully build broken due to proxy redirect issues, I was very happy today discovering we can control the underlying mechanism of rendering the pages to some degree.
Configure Proxy Redirect with Scully - The Static Site Generator for Angular apps
How I Used Ionic 4 CLI Proxy To Redirect API Requests And Avoid CORS Errors
I recently refactored an Ionic Angular app that previously made use of jQuery to fetch data and update the view 😔. Having moved the API calls into dedicated services, all API requests were blocked by the browser given the different origins (localhost vs external api url).
Angular: Dynamically Create a Div, Give it an ID, and Append it to the Body Element
Here’s how to dynamically create a div
, set it’s id
property, and append it to the body
element in an Angular service or component. I’ll use the example of creating a recaptcha container div on the fly.
Passing Additional Parameters To An Angular Service
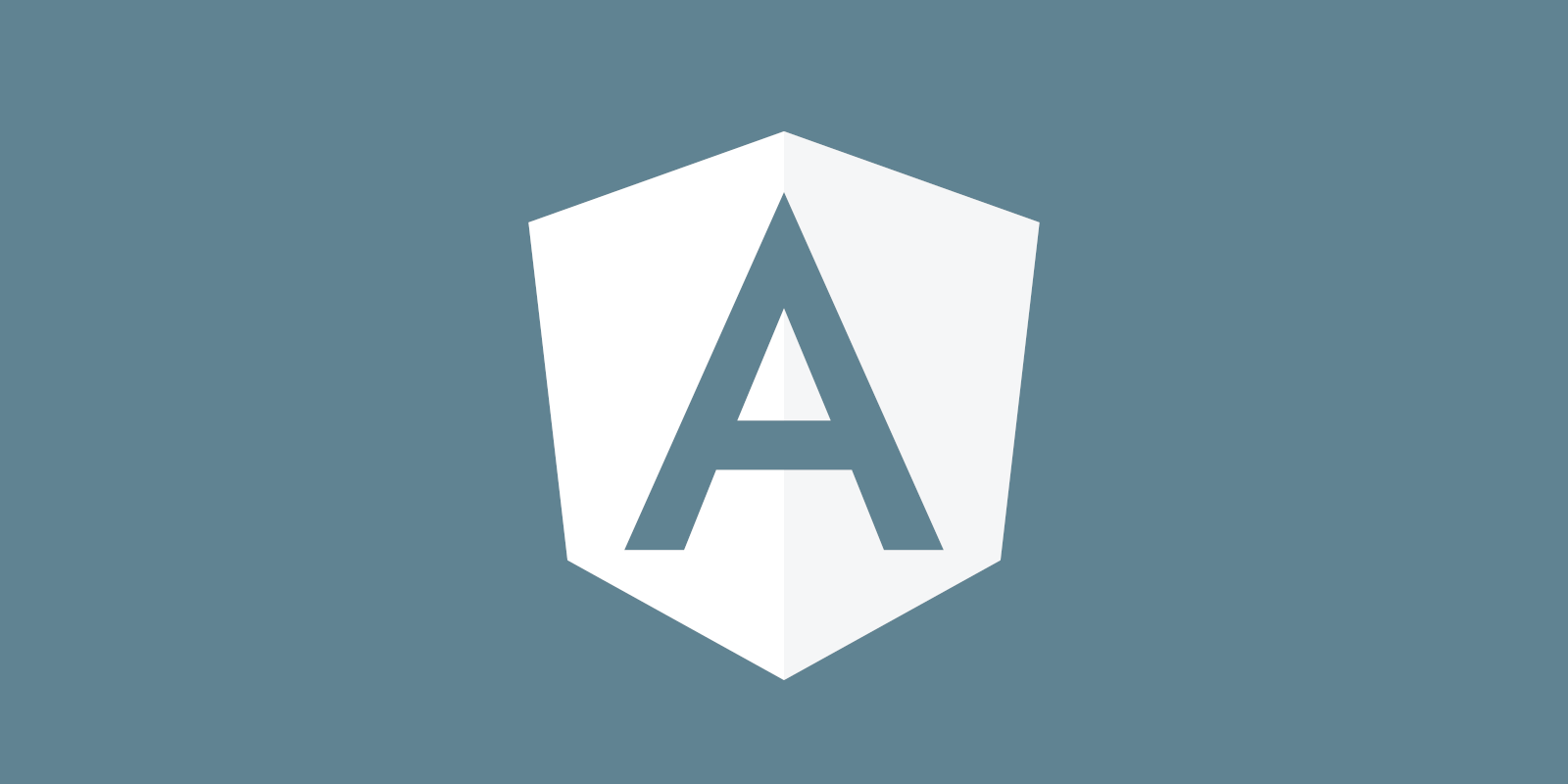
If you want to pass additional parameters to an Angular service, what you are looking for is @Inject decorator. It helps you pass your parameters to the service through Angular’s dependency injection mechanism.
Adding Custom Content To Akveo’s @nebular/theme 4.5.0 Sidebar Component
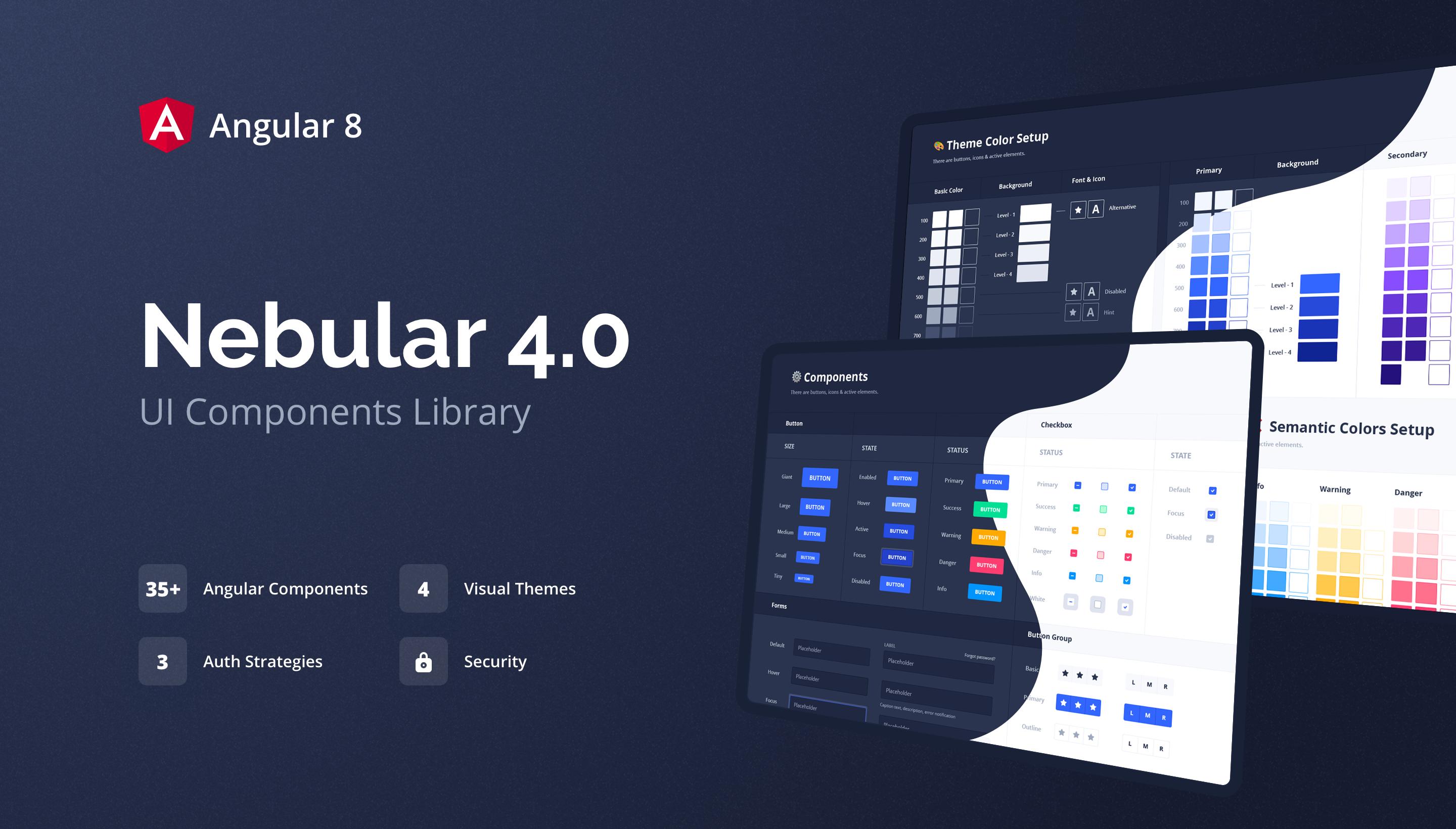
So I’ve been working with Akveo’s Nebular UI Kit for a while now and it’s been a real issue adding custom content to nb-sidebar
.
Angular: Allow Users Retry Failed Requests With A Twitter-Like Try Again Button
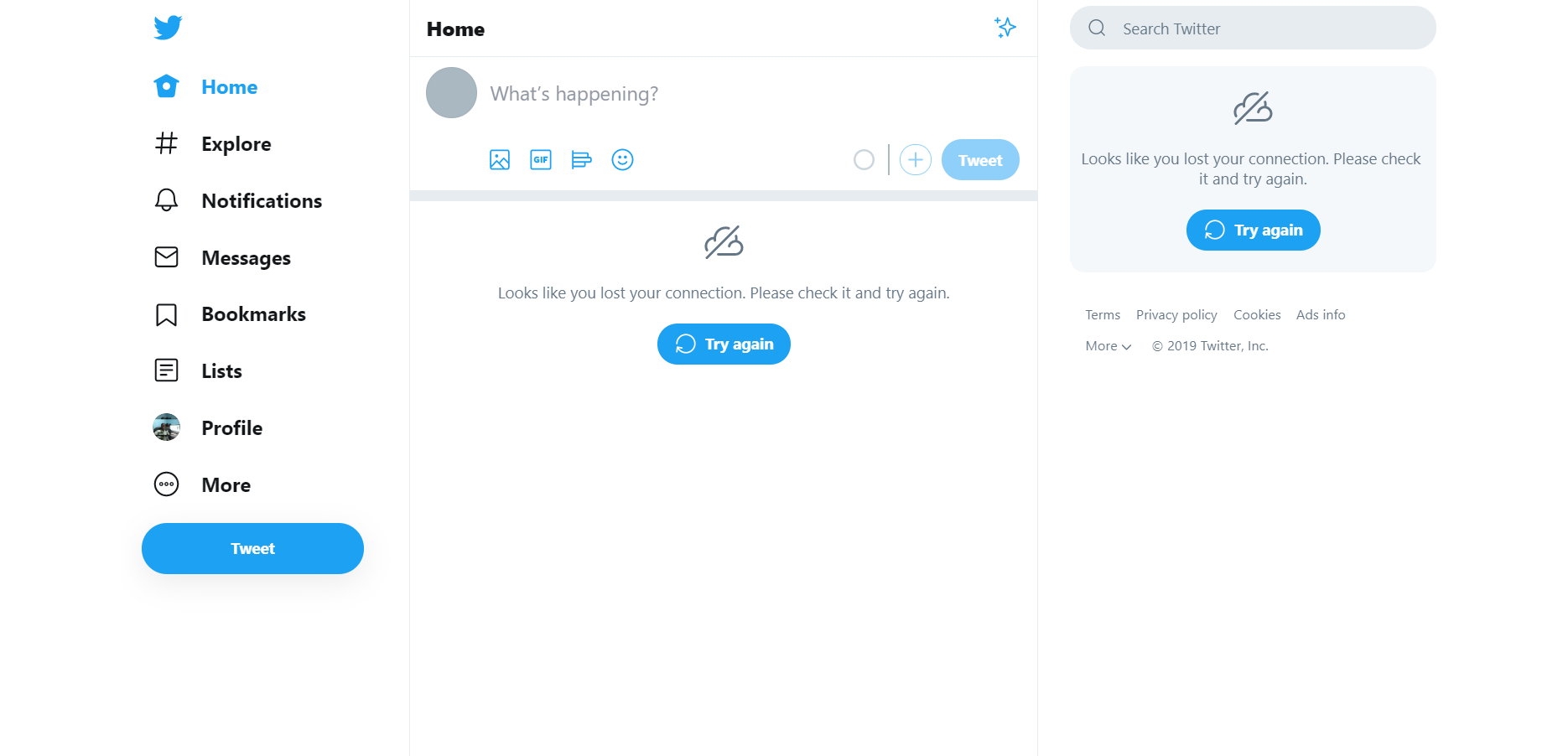
While using the Twitter web app, I noticed it displays a Try Again
button for failed requests in different sections of the user interface. This allows the user retry each failed request without affecting the rest of the application, quite neat.
Angular Tip: Hide Proxy Redirect CLI Command In angular.json
Wouldn’t it be great if instead of doing ng serve --proxy-config proxy.conf.js
you simply use ng serve
and the Angular CLI takes care of the proxy redirect command? Well, you can.
How Angular’s HttpErrorResponse is Created, What’s It Made Of?
This is mostly pulled from this article I recently read on Angular Http Error Handling, full credits to it’s original author TEKTUTORIALSHUB.
4 Angular NgForOf Exported Values I Was Not Aware Of - first, last, even, and odd
Angular’s NgForOf provides exported values that can be aliased to local variables. Until today, I’ve only been aware of the index
exported value, turns out there’s a couple more.
How To Extract and Display Laravel Validation Errors in Angular
Deep Cloning Objects in Angular, Typescript, Javascript
Some of the techniques for cloning objects in Javascript are either using object destructuring or a combination of JSON.stringify() and JSON.parse().
Setting Nebular Modal Size: My Approach
I’ve had this longstanding issue with setting the size of modals created with NbDialogService.
Test Angular Build Locally
To test your Angular build locally:
How to get a Reference to the Window Object in an Angular 8 Application
There are many articles on the web showing various methods of getting a reference to the window object in Angular (primarily through the dependency injection mechanism). However those that are popular on Google search are from 2016, 2017, etc, and the methods are mostly overly complicated (understandably).
Using the Url as the Single Source of Truth in an Angular Application
Lately i’ve been trying to apply the concept of using the url as the single source of truth in Angular applications I work on. Today I wrote some functionality that demonstrates this quite well.
Using the html <time></time> element with Angular
<time [dateTime]="'2019-08-09 16:22:20'">8/9/2019</time>
Painless Strongly Typed Angular Reactive Forms
A few days back while looking into strongly typing reactive forms in Angular, I came across this post by Alex Klaus. Given reactive forms don’t currently support strong typing (see issues #13721 and #17000), he suggests making use of Daniele Morosinotto solution which involves leveraging Typescript declaration files (*.d.ts
) .
Fix: Angular Route Resolver Receiving Data But Still Preventing Navigation To Route
Ever had this issue where for some reason you can’t navigate to a route even though everything seems ok, and the route’s data resolvers are all executing?
Attach agGrid Checkbox Selection to the First Displayed Column
From the agGrid documentation,
Straightforward Guide to Adding Bootstrap 4 Styles in an Angular Project
FYI, this is a to the point guide on adding and Bootstrap 4 styles (scss) to an Angular project.
Angular 2+ PDF Generation with jsPDF - Sample Project
I may flesh this out with a detailed post later, but if you have any questions or encounter any issues feel free to reach out to me.
Automatically Add a Protocol to a Url String in Angular
This little utility function can help you easily determine if a url string contains a protocol and add one if missing.
Using RXJS’s shareReplay() Operator to Prevent Firing New HTTP Requests for Template Subscriptions
Just read this article by Nicholas Jamieson which gave me a whole lot of new insights into how RxJs operator functions are written. I encourage you to have a look.
Fetching the Current Route Fragment in Angular 7
I’ve spent a few hours trying to get fragment navigation working properly for a use case on Xamcademy (https://xamcademy.com).
Using a directive to render Google Maps directions in Angular
A few months back while working on a location based taxi calling app, I had to build in functionality to render the route from the user’s location to the selected or searched listing on the map.
Angular 2+ Google Places Autocomplete directive
```typescript
///
Why you should use trackBy with Angular’s *ngFor loop
trackBy is a function which will return a unique identifier for each item in the array provided to *ngFor.
Normally when the array changes, Angular re-renders the whole DOM tree. But if you use trackBy, Angular will know which element has changed and will only make DOM changes for that particular element.
Using a base ControlValueAccessor class to quickly bootstrap Angular custom form controls
Having created a good number of custom form controls in Angular, it always felt repetitive implementing the ControlValueAccessor interface in each custom component.
Laravel
Laravel HTTP Client Retry Without Throwing an Exception
I used Laravel’s HTTP Client retry()
feature for the first time yesterday and was surprised it throws an exception on failure of all retries instead of returning the failure Illuminate\Http\Client\Response
object.
Laravel: Only retrieve a specific property from the first matching model
Using Laravel Eloquent, I wanted to only get a specific property - the id
- from the first matching model of my query.
Laravel: Dynamic Array Input Form Field Example
In my app, I have a promotion’s table which has a field called phone_restrictions
, which is an array of phone numbers a promotion is restricted to.
Laravel: Localized Carbon Date String Example
I wanted to return a translated date string from a Carbon date object and after some research, used the below solution which worked for me. It is quite brief, do leave a comment if you’ve got a question.
Laravel: Test replacement value is passed to validation string
Below is the validation language line we want to test, I added it to the array in resources/lang/en/validation.php
.
Laravel Validation: sometimes vs nullable
Source Code of Laravel Framework’s Validation Rules
If you’d like to view the source code of Laravel’s validation rules on Github, have a look here Validator.php#L1102.
After a long search through the Laravel Github repositories, I finally had a break and found the link through this Stackoverflow answer. I wanted to see how the framework actually validates integers.
Using Spatie’s Laravel Dashboard within an existing Page Layout
I’ve recently added Spatie’s Laravel Dashboard to a backend admin app and didn’t realize it is configured as a full webpage already, with an opening <html>
tag, <head>
, and <body>
all setup.
Format Dates for Humans with Carbon in PHP
I’ve spent like the last 30 minutes searching the web for how to convert dates to human-readable strings with Carbon in PHP/Laravel. Here’s a compilation of a couple of useful Carbon methods for my future self.
Format Date Column in Infyom Datatable - Laravel
I have the following datatable class generated by Infyom’s Laravel Generator:
Unit Testing Translation Strings in Laravel
If your Laravel app uses multiple locales, it can get tedious keeping track of translations that are yet to be added to the appropriate translation files, e.g English translations in resources/lang/en/validation.php
and French translations in resources/lang/fr/validation.php
.
Laravel Middleware to Set App Locale to Passed In Value
This is a middleware that takes care of setting the app locale to the passed in locale string (e.g en
, fr
).
How to access \App\Http\Kernel class instance in Laravel
To access an instance of your app’s App\Http\Kernel
class, you can use Laravel’s built in resolve()
helper function:
Pass Value to Replace a Custom Placeholder in a Laravel Validation Message
Say you have the following validation message in the custom
array of your language file - resources/lang/xx/validation.php
- (or another location depending on your use case).
Laravel: Exclude a Dynamic Route From CSRF Protection
Let’s say you have the following dynamic web route and would like to exclude it from csrf protection in your Laravel application:
Pass Typescript-like Typed Objects and Arrays to Your Laravel Functions by Leveraging Data Transfer Objects
When working on a PHP codebase, one thing I really miss from the Typescript world is the ability to pass properly typed objects and array into functions.
How To Extract and Display Laravel Validation Errors in Angular
Using Laravel’s Default Password Reset Functionality from an API - Sending the Notification
This assumes you’ve already setup API authentication in your Laravel application.
PHP
Safely Ignore Platform Requirements using Composer v2
I’ve learned the hard way that blindly using Composer’s --ignore-platform-reqs
can lead to issues like the downloading of package versions that are not compatible with your PHP version. I am on PHP 7.4 but packages using PHP 8 features were being downloaded, leading to this error:
Create a Seconds-Only PHP DateInterval
I wanted to test an interval was being respected which required me to add a seconds-based interval value to a Carbon date object.
Source Code of Laravel Framework’s Validation Rules
If you’d like to view the source code of Laravel’s validation rules on Github, have a look here Validator.php#L1102.
After a long search through the Laravel Github repositories, I finally had a break and found the link through this Stackoverflow answer. I wanted to see how the framework actually validates integers.
Using Spatie’s Laravel Dashboard within an existing Page Layout
I’ve recently added Spatie’s Laravel Dashboard to a backend admin app and didn’t realize it is configured as a full webpage already, with an opening <html>
tag, <head>
, and <body>
all setup.
How to Set Nullable Types in PHP >= 7.1.x
To set a function parameter or return type as nullable, add a question mark in front of the type declaration.
Format Dates for Humans with Carbon in PHP
I’ve spent like the last 30 minutes searching the web for how to convert dates to human-readable strings with Carbon in PHP/Laravel. Here’s a compilation of a couple of useful Carbon methods for my future self.
Format Date Column in Infyom Datatable - Laravel
I have the following datatable class generated by Infyom’s Laravel Generator:
Detecting CAMTEL Numbers with Regex
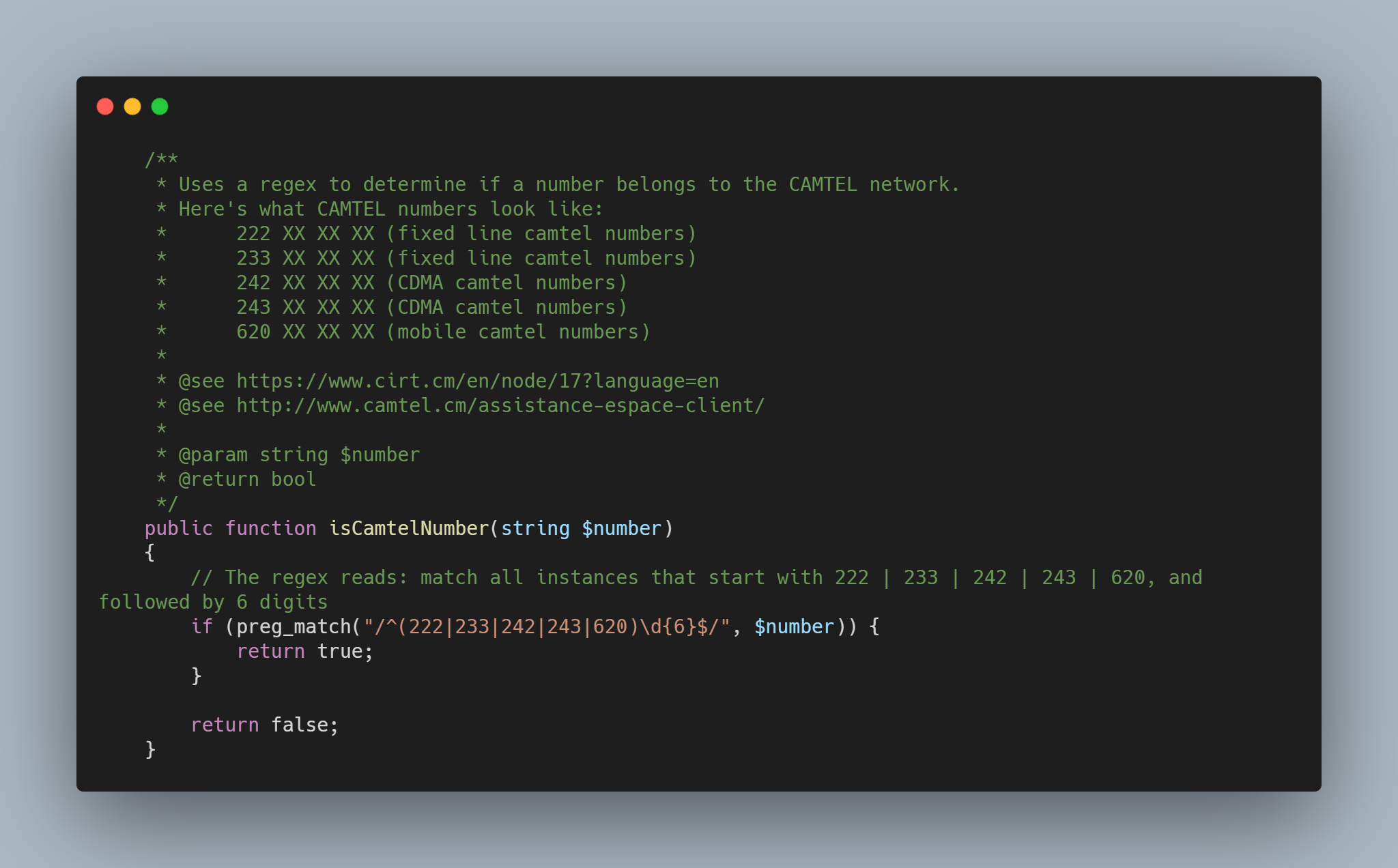
```php <?php
Pass Value to Replace a Custom Placeholder in a Laravel Validation Message
Say you have the following validation message in the custom
array of your language file - resources/lang/xx/validation.php
- (or another location depending on your use case).
Generating Unique Phone Numbers for Testing Purposes
Pass Typescript-like Typed Objects and Arrays to Your Laravel Functions by Leveraging Data Transfer Objects
When working on a PHP codebase, one thing I really miss from the Typescript world is the ability to pass properly typed objects and array into functions.
Detect The Carrier/Mobile Network Of A Phone Number With Javascript, PHP, Java, C++, Ruby, etc
For a long time I’ve struggled with this problem, determining the Mobile Network a given phone number belongs to. Today I found out Google’s libphonenumber library (or any of it’s third party ports for other languages) have this functionality built in.
Featured
An in-depth look at RxJS’s distinctUntilChanged Operator
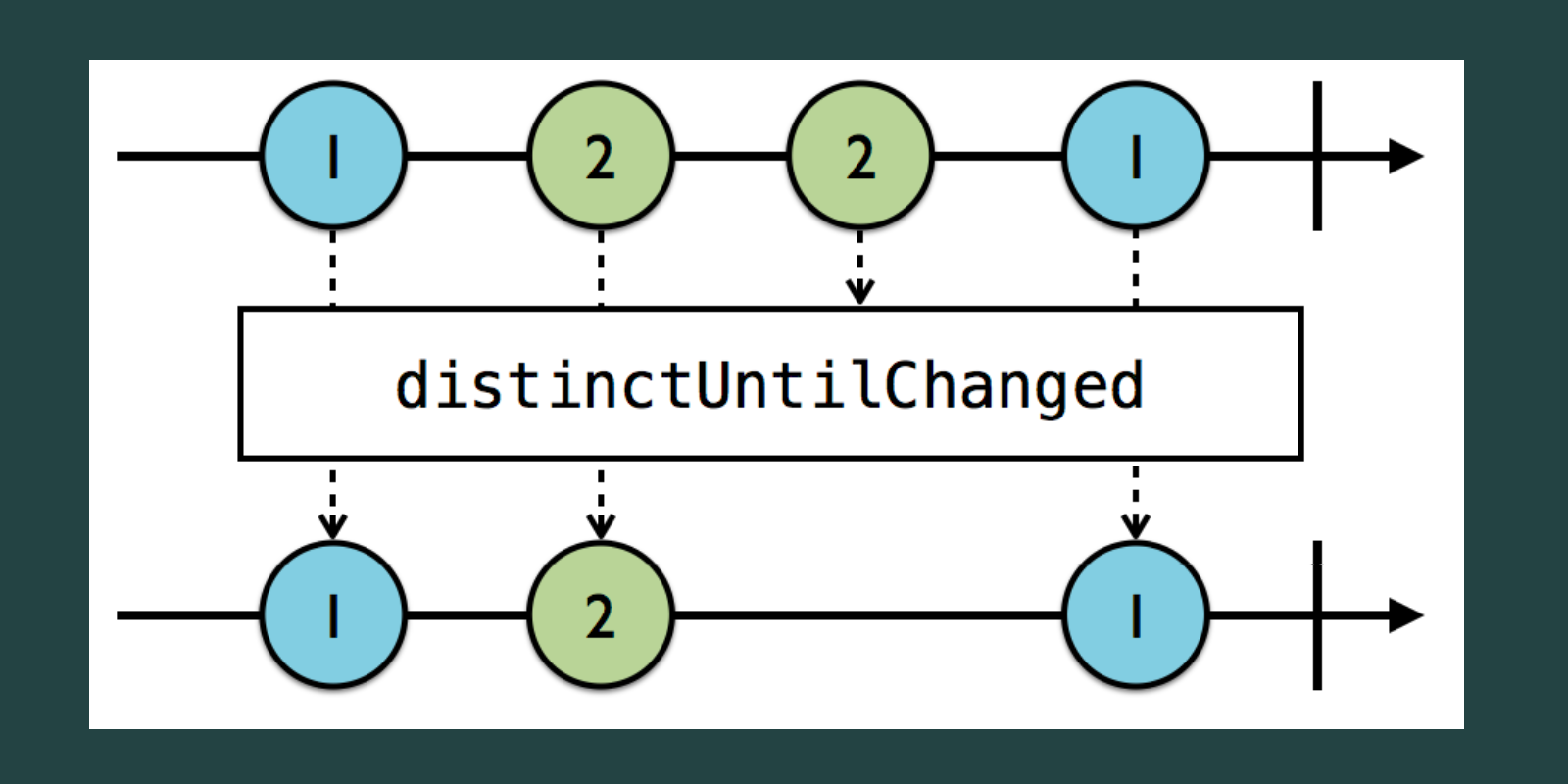
In this post, we’ll have an in-depth look at RxJS’s distinctUntilChanged
operator, it’s signature and what it does, it’s parameters compare
and keySelector
, and typical use cases for each of them and both of them.
Creating a Delayed Input Directive in Angular
Imagine in your app there’s a search input that triggers an http request on each keystroke as a user types in their query. As your userbase grows, search operations quickly become expensive due to the increased traffic to your server.
Creating a Lazy Loaded Image Cropping Modal Component with Ionic 5 and ngx-image-cropper
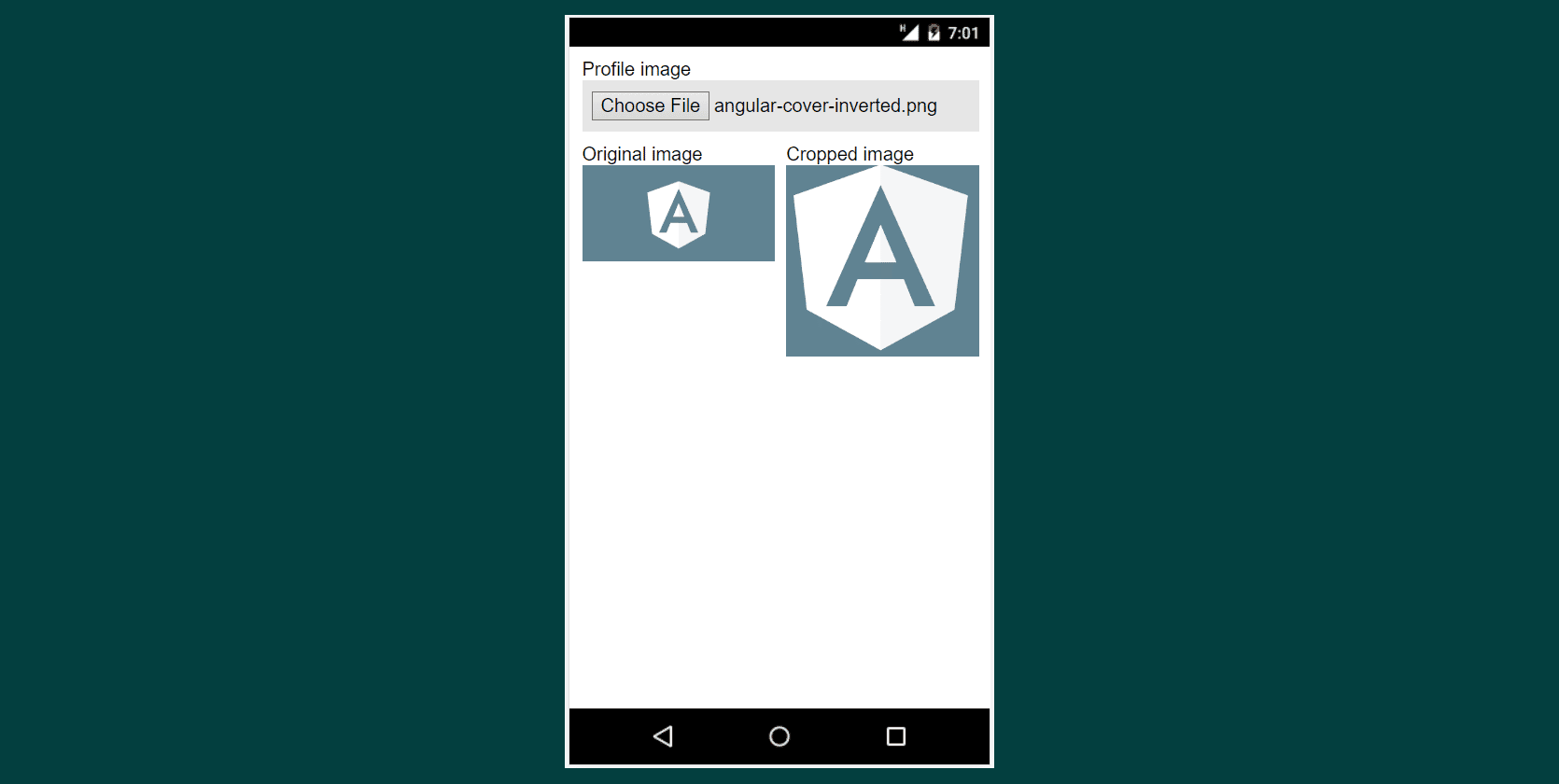
Demo and source code
Configure Proxy Redirect with Scully - The Static Site Generator for Angular apps
Passing Additional Parameters To An Angular Service
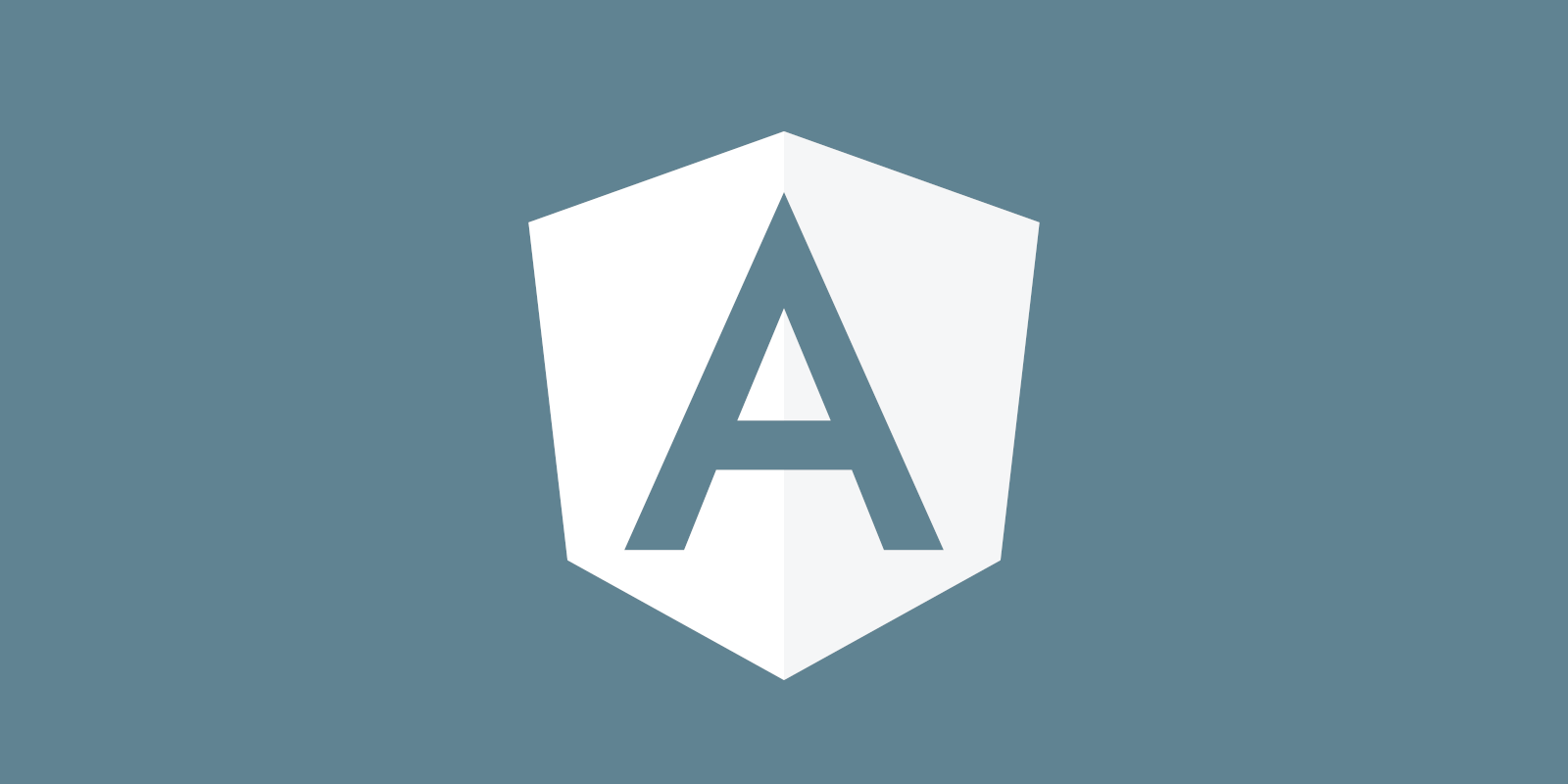
If you want to pass additional parameters to an Angular service, what you are looking for is @Inject decorator. It helps you pass your parameters to the service through Angular’s dependency injection mechanism.
Adding Custom Content To Akveo’s @nebular/theme 4.5.0 Sidebar Component
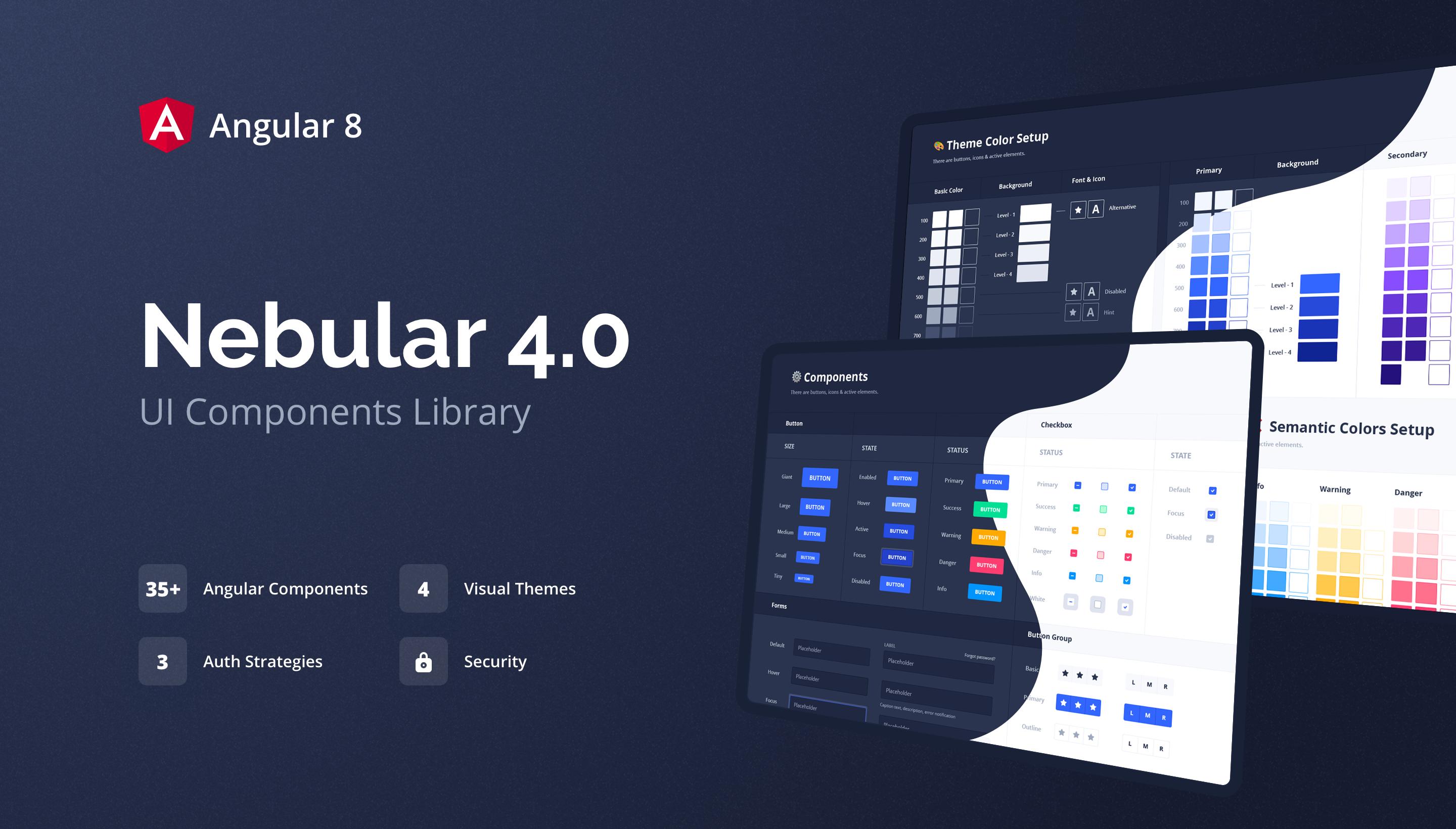
So I’ve been working with Akveo’s Nebular UI Kit for a while now and it’s been a real issue adding custom content to nb-sidebar
.
Angular: Allow Users Retry Failed Requests With A Twitter-Like Try Again Button
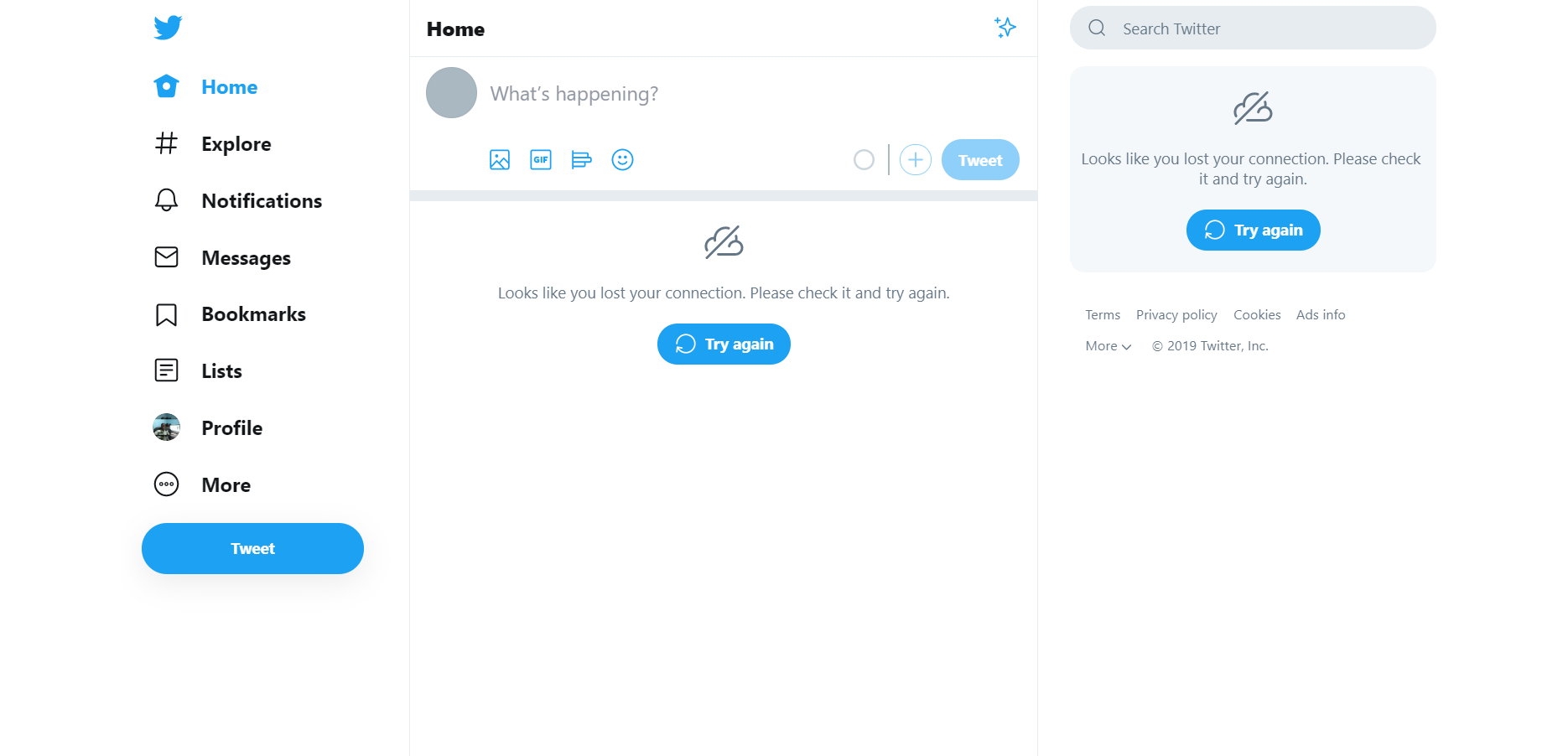
While using the Twitter web app, I noticed it displays a Try Again
button for failed requests in different sections of the user interface. This allows the user retry each failed request without affecting the rest of the application, quite neat.
4 Angular NgForOf Exported Values I Was Not Aware Of - first, last, even, and odd
Angular’s NgForOf provides exported values that can be aliased to local variables. Until today, I’ve only been aware of the index
exported value, turns out there’s a couple more.
How To Extract and Display Laravel Validation Errors in Angular
Ionic
Netlify build command for an Ionic Angular app
In the Build command
input field, enter ng build --prod
, and in the Publish directory
input field, enter www
.
Test an Ionic Build, or an Ionic PWA locally
If you don’t have http-server
installed, go ahead and install it globally.
Rendering ion-item’s Content “Predictably”
<ion-item>CONTENT</ion-item>
Exploring Ionic Image Preloading Scenarios with ionic-image-loader
ionic-image-loader is an Ionic 2+ component that loads images in a background thread and caches them for later use. It also makes available a service to manually preload images, which will be the focus of this post.
Ionic 4 Swipe Navigation Between Pages
Imagine in your app there’s a page with a list of items, and clicking on an item navigates to and item details page. On the details page, you’d like to be able to swipe left to bring up the previous item, or right to bring up the next item. This post aims to provide an implementation for such functionality.
Creating a Lazy Loaded Image Cropping Modal Component with Ionic 5 and ngx-image-cropper
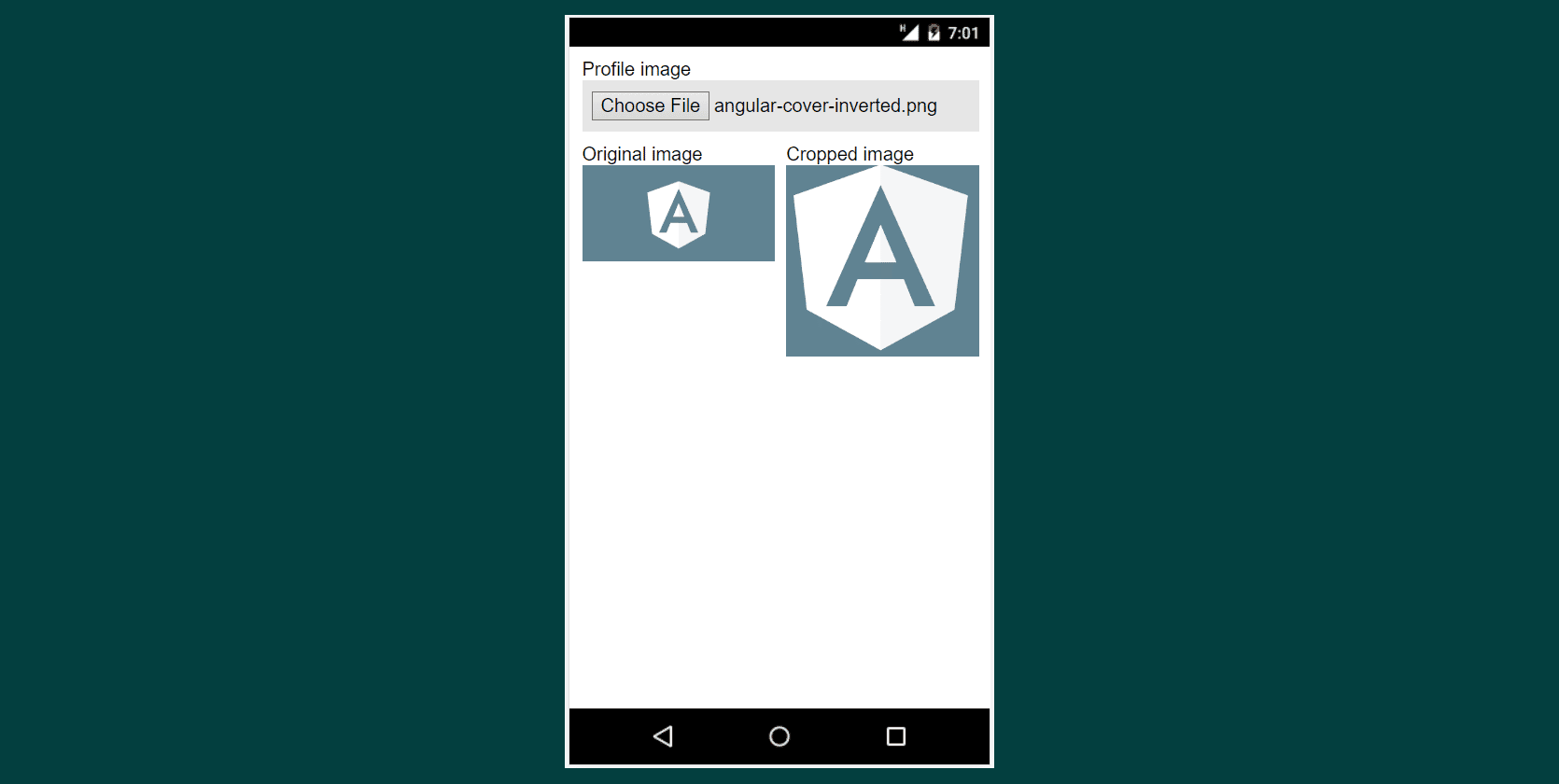
Demo and source code
How I Used Ionic 4 CLI Proxy To Redirect API Requests And Avoid CORS Errors
I recently refactored an Ionic Angular app that previously made use of jQuery to fetch data and update the view 😔. Having moved the API calls into dedicated services, all API requests were blocked by the browser given the different origins (localhost vs external api url).
Javascript
Run an npm script in package.json inside another npm script in package.json
This post might seem trivial but I did spend a good amount of time searching this online just to be sure. So here we are lol.
You can pass a callback function to RxJS’s first() operator, I was not aware!
My typical use-case for RxJS first() operator is to emit only the first item of an observable stream. What if you want the first value based on a certain condition instead?
Detect The Carrier/Mobile Network Of A Phone Number With Javascript, PHP, Java, C++, Ruby, etc
For a long time I’ve struggled with this problem, determining the Mobile Network a given phone number belongs to. Today I found out Google’s libphonenumber library (or any of it’s third party ports for other languages) have this functionality built in.
Deep Cloning Objects in Angular, Typescript, Javascript
Some of the techniques for cloning objects in Javascript are either using object destructuring or a combination of JSON.stringify() and JSON.parse().
On Exception Handling with try/catch in Javascript
Early today was researching why I don’t see a lot of exception handling with try/catch in Javascript codebases.
Format and Display a Javascript Date Object as Date and Time
Say we have the following Javascript date object and would like to display it as date and time.
Conditionally Add a Member to an Object in Javascript Using the Spread Operator
Say you have an object you’d like to conditionally add a member to, here’s a neat little trick to do it with the spread operator.
Jekyll
Add Support for HTML5 Video Tag to a Jekyll Blog
You can use one of the below approaches:
- Use the Octopress plugin as described in this Stackoverflow answer.
- Use the approach in this pull request to the Jekyll So Simple Theme. You may have to update the code to match your video storage path.
Specify a default value for Netlify CMS string widget
To specify a default value for Netlify CMS’s string
widget, add the default
property to it’s definition and assign it a value, as follows:
Resources for Syntax Highlighting in Jekyll with Rouge
Here’s the best resources I’ve found for configuring syntax highlight with Route in Jekyll:
Jekyll: Setting a default cover image for all your posts
This post assumes you’re currently able to add an image
to your post front matter, for example:
Blogging
Specify a default value for Netlify CMS string widget
To specify a default value for Netlify CMS’s string
widget, add the default
property to it’s definition and assign it a value, as follows:
Resources for Syntax Highlighting in Jekyll with Rouge
Here’s the best resources I’ve found for configuring syntax highlight with Route in Jekyll:
Jekyll: Setting a default cover image for all your posts
This post assumes you’re currently able to add an image
to your post front matter, for example:
Typescript
Pass Typescript-like Typed Objects and Arrays to Your Laravel Functions by Leveraging Data Transfer Objects
When working on a PHP codebase, one thing I really miss from the Typescript world is the ability to pass properly typed objects and array into functions.
Generating Typescript Interfaces Directly From The Database
The past couple of weeks I’ve been adding new functionality to an API and today began updating the Angular front-end application to reflect these changes.
Deep Cloning Objects in Angular, Typescript, Javascript
Some of the techniques for cloning objects in Javascript are either using object destructuring or a combination of JSON.stringify() and JSON.parse().
*ngFor
4 Angular NgForOf Exported Values I Was Not Aware Of - first, last, even, and odd
Angular’s NgForOf provides exported values that can be aliased to local variables. Until today, I’ve only been aware of the index
exported value, turns out there’s a couple more.
Why you should use trackBy with Angular’s *ngFor loop
trackBy is a function which will return a unique identifier for each item in the array provided to *ngFor.
Normally when the array changes, Angular re-renders the whole DOM tree. But if you use trackBy, Angular will know which element has changed and will only make DOM changes for that particular element.
Angular CLI
Angular Tip: Hide Proxy Redirect CLI Command In angular.json
Wouldn’t it be great if instead of doing ng serve --proxy-config proxy.conf.js
you simply use ng serve
and the Angular CLI takes care of the proxy redirect command? Well, you can.
Test Angular Build Locally
To test your Angular build locally:
Cypress
Two Ways to get a Form Input’s Value in Cypress
Given the below form, how do we get any individual input’s value inside a Cypress test?
Testing File Download Links with Cypress
Say my homepage contains the below snippet, which allows a user to download vcard files. I’d like to make sure the file download links are valid and point to existing files.
Mapbox
Determine Mapbox map tiles have loaded by listening for the “idle” event
The idle
event is fired after the last frame rendered before the map enters an “idle” state. When this event is fired, we are sure of the following things:
- All currently requested tiles have loaded.
- No camera transitions are in progress.
- All fade/transition animations have completed.
Angular: Conditionally Rendering Mapbox Markers
Say we’re using Mapbox to render a map that contains a couple of icons representing places. When the map loads for the first time, no marker should be rendered.
Netlify
Netlify build command for an Ionic Angular app
In the Build command
input field, enter ng build --prod
, and in the Publish directory
input field, enter www
.
Netlify: Redirect Users by Country or Language
Today at Switchn we had the need to redirect users by country and browser language on our Netlify hosted site. That is, send those visiting our site from English speaking countries to /en/
and those from French speaking countries to /fr/
. Also if the user’s browser sends an accept-language
header in the request (e.g. accept-language: en-US,en;q=0.9
), we’d like to take that into consideration too.
PWA
Test an Ionic Build, or an Ionic PWA locally
If you don’t have http-server
installed, go ahead and install it globally.
Angular Service Worker: Prefetch all assets on first run
I’ve been working on the PWA version of a mobile app and it was brought to my attention that when using the PWA offline, some icons that were placed in the /src/assets
directory fail to load.
RxJS
An in-depth look at RxJS’s distinctUntilChanged Operator
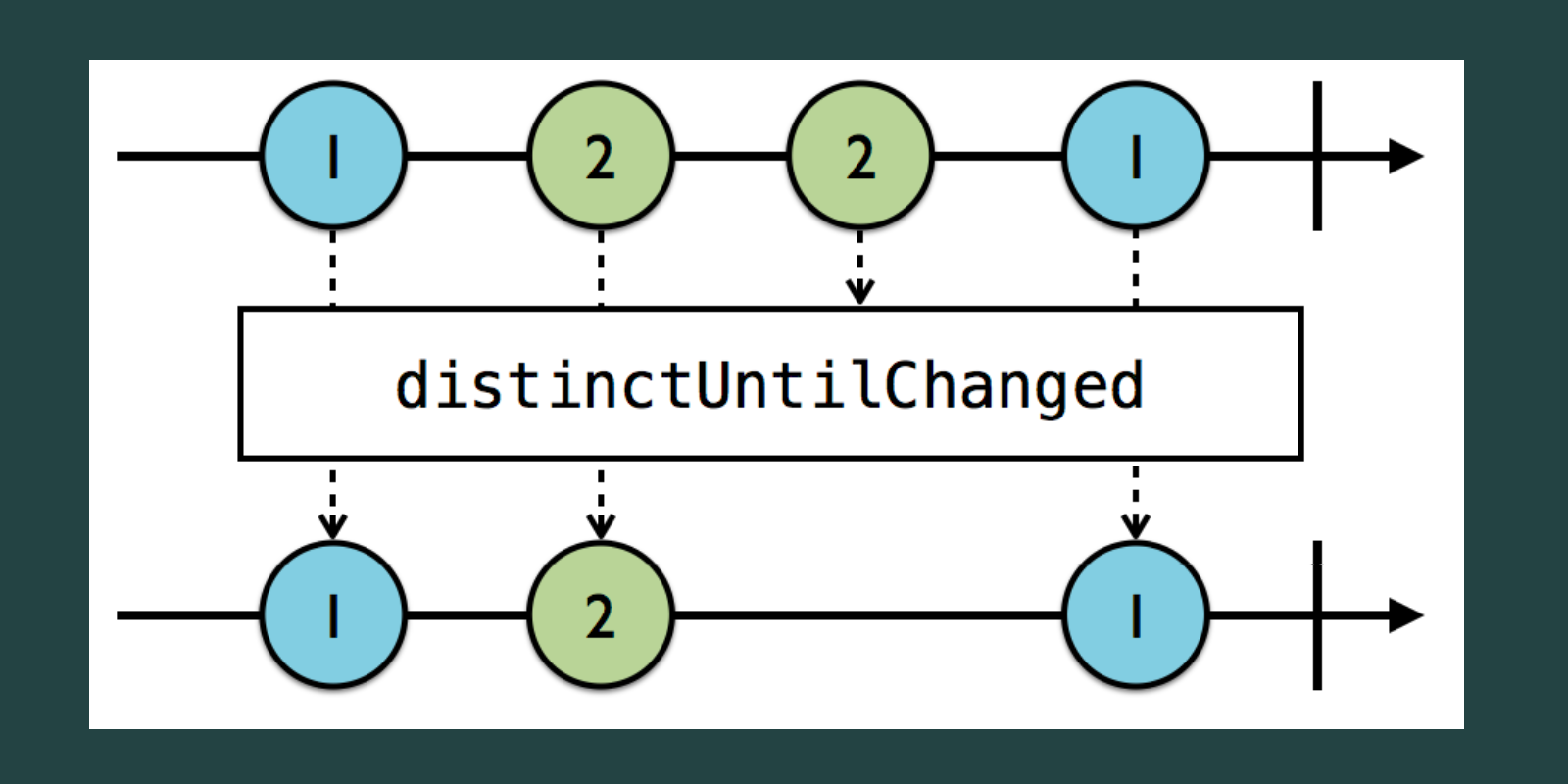
In this post, we’ll have an in-depth look at RxJS’s distinctUntilChanged
operator, it’s signature and what it does, it’s parameters compare
and keySelector
, and typical use cases for each of them and both of them.
You can pass a callback function to RxJS’s first() operator, I was not aware!
My typical use-case for RxJS first() operator is to emit only the first item of an observable stream. What if you want the first value based on a certain condition instead?
ScullyIO
Debugging ScullyIO Rendering and Network Errors with the help of puppeteerLaunchOptions
With my Angular app’s Scully build broken due to proxy redirect issues, I was very happy today discovering we can control the underlying mechanism of rendering the pages to some degree.
Configure Proxy Redirect with Scully - The Static Site Generator for Angular apps
Unit Testing
Laravel: Test replacement value is passed to validation string
Below is the validation language line we want to test, I added it to the array in resources/lang/en/validation.php
.
Unit Testing Translation Strings in Laravel
If your Laravel app uses multiple locales, it can get tedious keeping track of translations that are yet to be added to the appropriate translation files, e.g English translations in resources/lang/en/validation.php
and French translations in resources/lang/fr/validation.php
.
AGM
Using a directive to render Google Maps directions in Angular
A few months back while working on a location based taxi calling app, I had to build in functionality to render the route from the user’s location to the selected or searched listing on the map.
API
Using Laravel’s Default Password Reset Functionality from an API - Sending the Notification
This assumes you’ve already setup API authentication in your Laravel application.
API Redirect
How I Used Ionic 4 CLI Proxy To Redirect API Requests And Avoid CORS Errors
I recently refactored an Ionic Angular app that previously made use of jQuery to fetch data and update the view 😔. Having moved the API calls into dedicated services, all API requests were blocked by the browser given the different origins (localhost vs external api url).
Automation
Generating Typescript Interfaces Directly From The Database
The past couple of weeks I’ve been adding new functionality to an API and today began updating the Angular front-end application to reflect these changes.
Bootstrap 4
Straightforward Guide to Adding Bootstrap 4 Styles in an Angular Project
FYI, this is a to the point guide on adding and Bootstrap 4 styles (scss) to an Angular project.
CAMTEL
Detecting CAMTEL Numbers with Regex
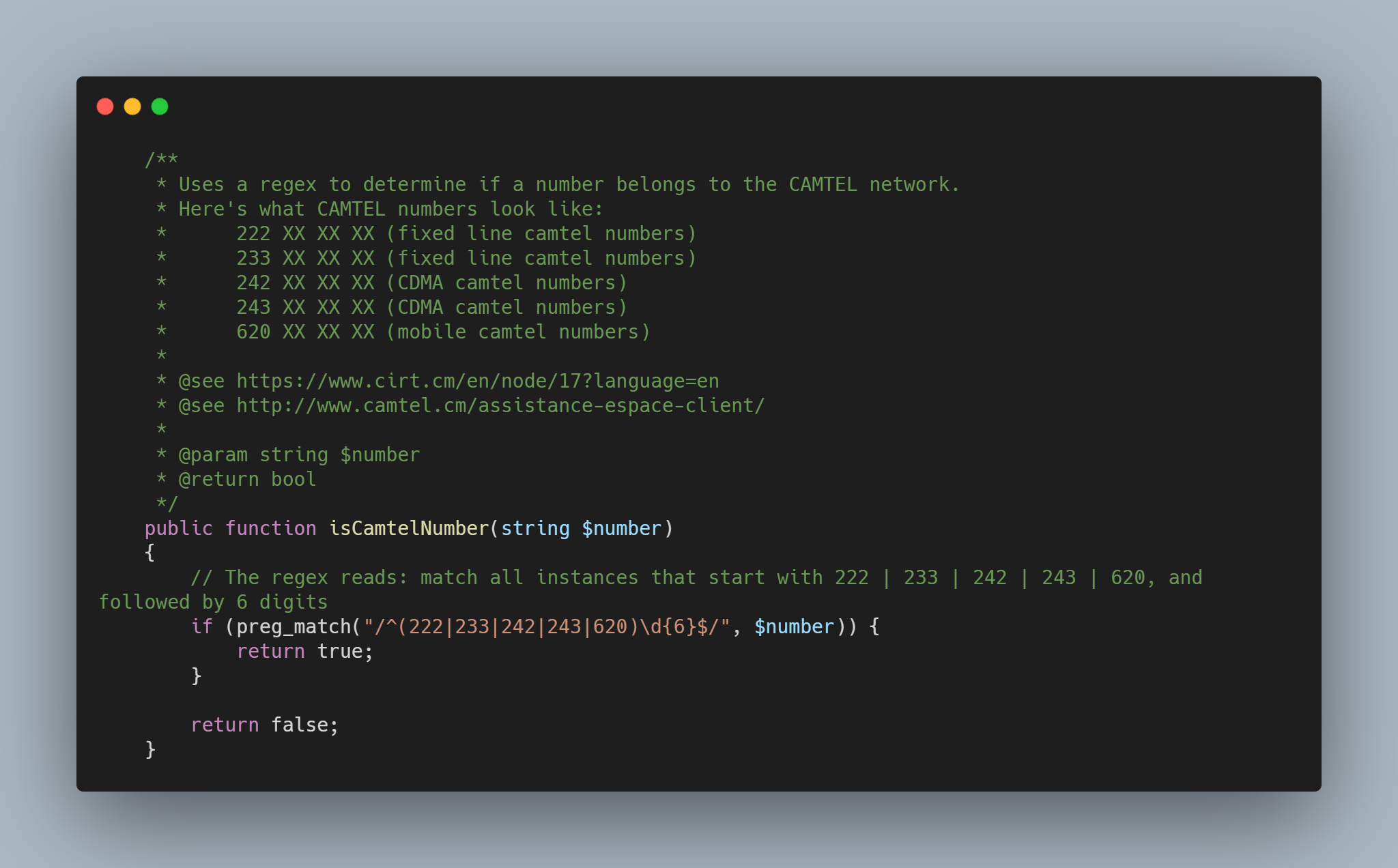
```php <?php
CSRF
Laravel: Exclude a Dynamic Route From CSRF Protection
Let’s say you have the following dynamic web route and would like to exclude it from csrf protection in your Laravel application:
Composer
Safely Ignore Platform Requirements using Composer v2
I’ve learned the hard way that blindly using Composer’s --ignore-platform-reqs
can lead to issues like the downloading of package versions that are not compatible with your PHP version. I am on PHP 7.4 but packages using PHP 8 features were being downloaded, leading to this error:
ControlValueAccessor
Using a base ControlValueAccessor class to quickly bootstrap Angular custom form controls
Having created a good number of custom form controls in Angular, it always felt repetitive implementing the ControlValueAccessor interface in each custom component.
Custom Form Control
Using a base ControlValueAccessor class to quickly bootstrap Angular custom form controls
Having created a good number of custom form controls in Angular, it always felt repetitive implementing the ControlValueAccessor interface in each custom component.
DTOs
Pass Typescript-like Typed Objects and Arrays to Your Laravel Functions by Leveraging Data Transfer Objects
When working on a PHP codebase, one thing I really miss from the Typescript world is the ability to pass properly typed objects and array into functions.
Databases
Generating Typescript Interfaces Directly From The Database
The past couple of weeks I’ve been adding new functionality to an API and today began updating the Angular front-end application to reflect these changes.
Date and Time Formatting
Format and Display a Javascript Date Object as Date and Time
Say we have the following Javascript date object and would like to display it as date and time.
DateInterval
Create a Seconds-Only PHP DateInterval
I wanted to test an interval was being respected which required me to add a seconds-based interval value to a Carbon date object.
Deep Cloning Objects
Deep Cloning Objects in Angular, Typescript, Javascript
Some of the techniques for cloning objects in Javascript are either using object destructuring or a combination of JSON.stringify() and JSON.parse().
Dependency Injection
Passing Additional Parameters To An Angular Service
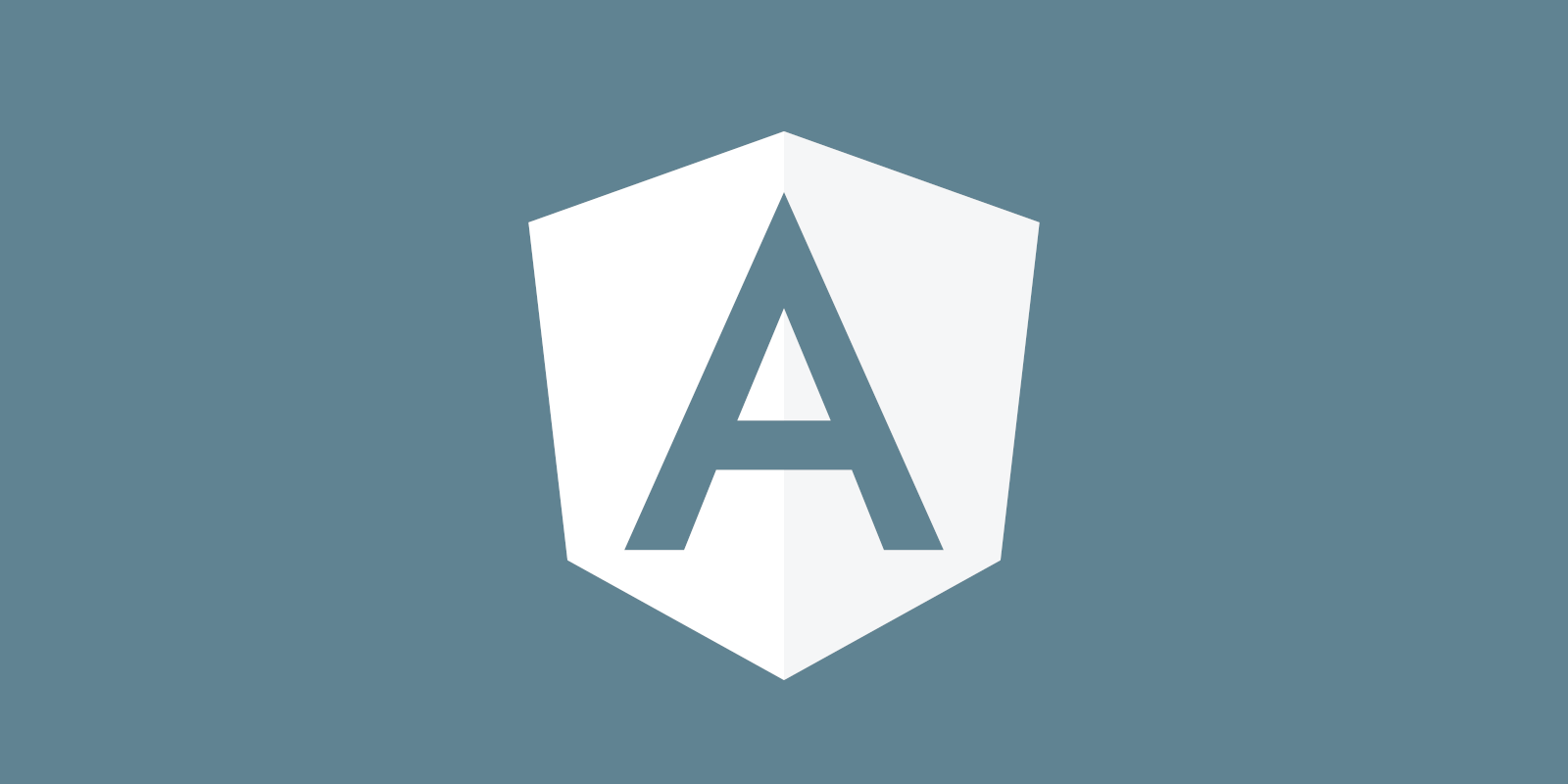
If you want to pass additional parameters to an Angular service, what you are looking for is @Inject decorator. It helps you pass your parameters to the service through Angular’s dependency injection mechanism.
Directions Renderer
Using a directive to render Google Maps directions in Angular
A few months back while working on a location based taxi calling app, I had to build in functionality to render the route from the user’s location to the selected or searched listing on the map.
Directions Service
Using a directive to render Google Maps directions in Angular
A few months back while working on a location based taxi calling app, I had to build in functionality to render the route from the user’s location to the selected or searched listing on the map.
Directive
Using a directive to render Google Maps directions in Angular
A few months back while working on a location based taxi calling app, I had to build in functionality to render the route from the user’s location to the selected or searched listing on the map.
Directives
Angular 2+ Google Places Autocomplete directive
```typescript
///
Exception Handling
On Exception Handling with try/catch in Javascript
Early today was researching why I don’t see a lot of exception handling with try/catch in Javascript codebases.
Exceptions
On Exception Handling with try/catch in Javascript
Early today was researching why I don’t see a lot of exception handling with try/catch in Javascript codebases.
Form Arrays
Using RXJS’s shareReplay() Operator to Prevent Firing New HTTP Requests for Template Subscriptions
Just read this article by Nicholas Jamieson which gave me a whole lot of new insights into how RxJs operator functions are written. I encourage you to have a look.
Google Maps
Using a directive to render Google Maps directions in Angular
A few months back while working on a location based taxi calling app, I had to build in functionality to render the route from the user’s location to the selected or searched listing on the map.
Google Places Autocomplete
Angular 2+ Google Places Autocomplete directive
```typescript
///
HttpErrorResponse
How Angular’s HttpErrorResponse is Created, What’s It Made Of?
This is mostly pulled from this article I recently read on Angular Http Error Handling, full credits to it’s original author TEKTUTORIALSHUB.
Injection Token
Passing Additional Parameters To An Angular Service
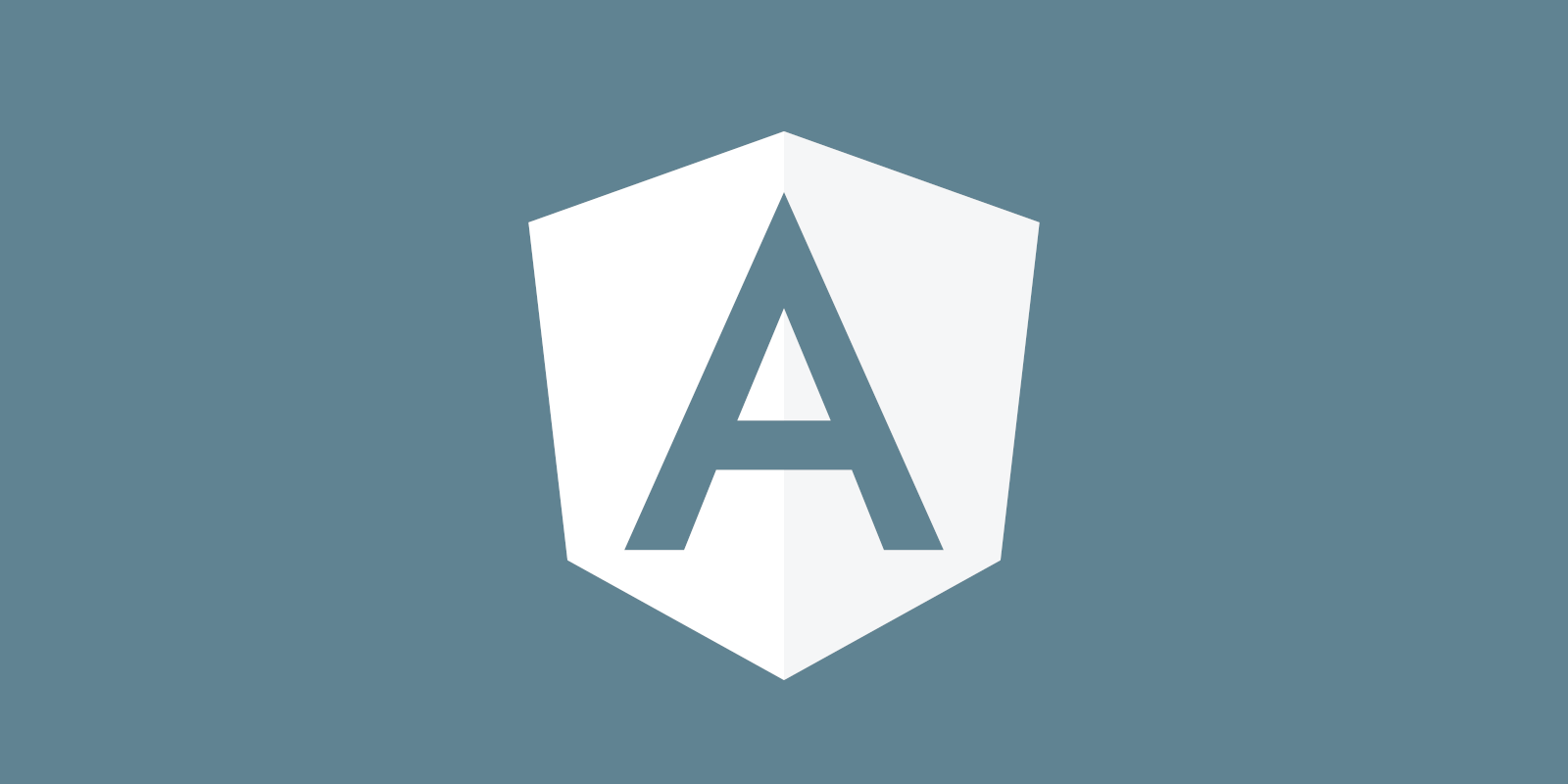
If you want to pass additional parameters to an Angular service, what you are looking for is @Inject decorator. It helps you pass your parameters to the service through Angular’s dependency injection mechanism.
Javascript Date Object
Format and Display a Javascript Date Object as Date and Time
Say we have the following Javascript date object and would like to display it as date and time.
KaiOS
Enable Adb Debug on MTN Smart T running KaiOS
To enable adb debug on MTN Smart T running KaiOS, dial this code to open a menu and from there enable the USB debugging:
Laravel Horizon
Install Laravel Horizon in Laravel 7
To install Laravel Horizon in Laravel 7, run below command in your terminal:
Laravel Validation Errors
How To Extract and Display Laravel Validation Errors in Angular
Livewire
Livewire: Confirm form submission before proceeding
Spent the last couple of hours trying to get this working. Here’s what finally worked:
MySQL
Storing timespans in a MySQL database
I’ve been working on coupon/promotion codes functionality and one requirement is only allowing the re-use of a coupon code after a period of time has passed. It could be a couple of hours, a couple of days, or a month at most.
NPM
Run an npm script in package.json inside another npm script in package.json
This post might seem trivial but I did spend a good amount of time searching this online just to be sure. So here we are lol.
NbDialogService
Setting Nebular Modal Size: My Approach
I’ve had this longstanding issue with setting the size of modals created with NbDialogService.
Nebular
Setting Nebular Modal Size: My Approach
I’ve had this longstanding issue with setting the size of modals created with NbDialogService.
Nebular UI Kit
Adding Custom Content To Akveo’s @nebular/theme 4.5.0 Sidebar Component
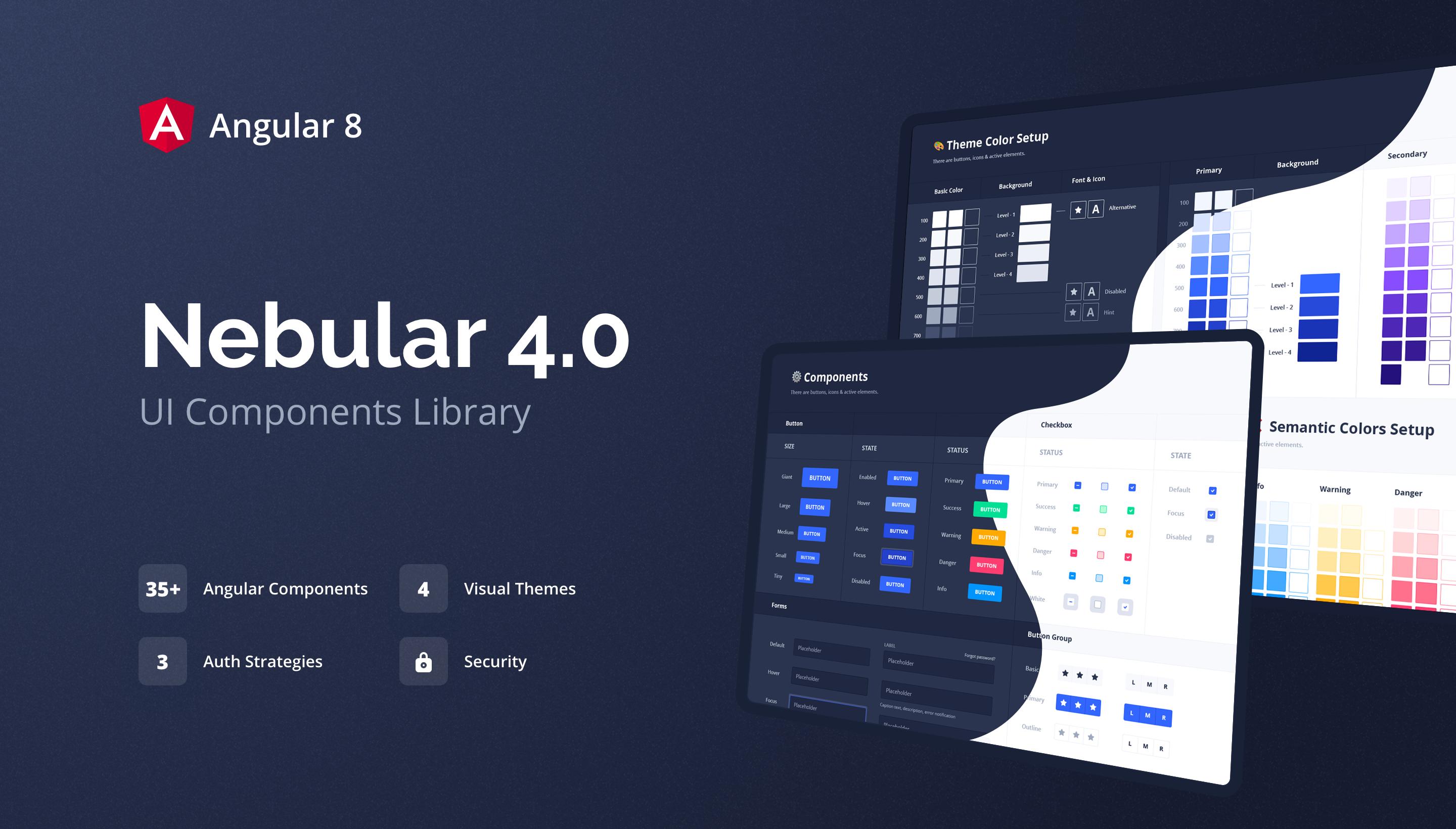
So I’ve been working with Akveo’s Nebular UI Kit for a while now and it’s been a real issue adding custom content to nb-sidebar
.
Netlify CMS
Specify a default value for Netlify CMS string widget
To specify a default value for Netlify CMS’s string
widget, add the default
property to it’s definition and assign it a value, as follows:
PDF Generation with Angular
Angular 2+ PDF Generation with jsPDF - Sample Project
I may flesh this out with a detailed post later, but if you have any questions or encounter any issues feel free to reach out to me.
Password Reset
Using Laravel’s Default Password Reset Functionality from an API - Sending the Notification
This assumes you’ve already setup API authentication in your Laravel application.
Proxy Redirect
Angular Tip: Hide Proxy Redirect CLI Command In angular.json
Wouldn’t it be great if instead of doing ng serve --proxy-config proxy.conf.js
you simply use ng serve
and the Angular CLI takes care of the proxy redirect command? Well, you can.
Reactive Forms
Painless Strongly Typed Angular Reactive Forms
A few days back while looking into strongly typing reactive forms in Angular, I came across this post by Alex Klaus. Given reactive forms don’t currently support strong typing (see issues #13721 and #17000), he suggests making use of Daniele Morosinotto solution which involves leveraging Typescript declaration files (*.d.ts
) .
Renderer2
Angular: Dynamically Create a Div, Give it an ID, and Append it to the Body Element
Here’s how to dynamically create a div
, set it’s id
property, and append it to the body
element in an Angular service or component. I’ll use the example of creating a recaptcha container div on the fly.
RendererFactory2
Angular: Dynamically Create a Div, Give it an ID, and Append it to the Body Element
Here’s how to dynamically create a div
, set it’s id
property, and append it to the body
element in an Angular service or component. I’ll use the example of creating a recaptcha container div on the fly.
Retry Failed Requests
Angular: Allow Users Retry Failed Requests With A Twitter-Like Try Again Button
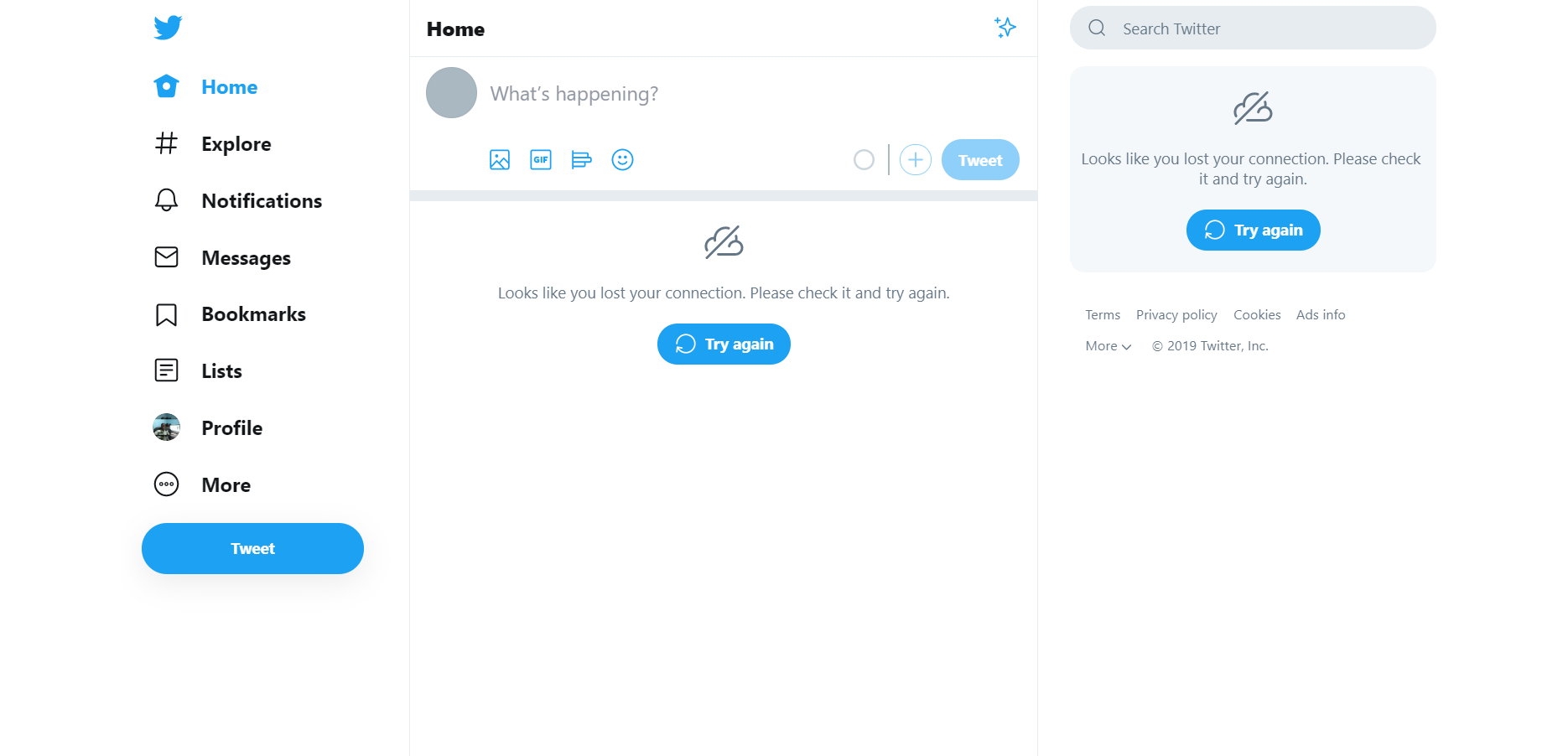
While using the Twitter web app, I noticed it displays a Try Again
button for failed requests in different sections of the user interface. This allows the user retry each failed request without affecting the rest of the application, quite neat.
Rouge
Resources for Syntax Highlighting in Jekyll with Rouge
Here’s the best resources I’ve found for configuring syntax highlight with Route in Jekyll:
Router Resolver
Fix: Angular Route Resolver Receiving Data But Still Preventing Navigation To Route
Ever had this issue where for some reason you can’t navigate to a route even though everything seems ok, and the route’s data resolvers are all executing?
RxJs
Using RXJS’s shareReplay() Operator to Prevent Firing New HTTP Requests for Template Subscriptions
Just read this article by Nicholas Jamieson which gave me a whole lot of new insights into how RxJs operator functions are written. I encourage you to have a look.
SCSS
Straightforward Guide to Adding Bootstrap 4 Styles in an Angular Project
FYI, this is a to the point guide on adding and Bootstrap 4 styles (scss) to an Angular project.
Spread Operator
Conditionally Add a Member to an Object in Javascript Using the Spread Operator
Say you have an object you’d like to conditionally add a member to, here’s a neat little trick to do it with the spread operator.
State Management
Using the Url as the Single Source of Truth in an Angular Application
Lately i’ve been trying to apply the concept of using the url as the single source of truth in Angular applications I work on. Today I wrote some functionality that demonstrates this quite well.
Strongly Typed Reactive Forms
Painless Strongly Typed Angular Reactive Forms
A few days back while looking into strongly typing reactive forms in Angular, I came across this post by Alex Klaus. Given reactive forms don’t currently support strong typing (see issues #13721 and #17000), he suggests making use of Daniele Morosinotto solution which involves leveraging Typescript declaration files (*.d.ts
) .
Testing
Back to Top ↑Text Processing
How YAML Helped Me Format and Process Text Data From Documents Much Faster
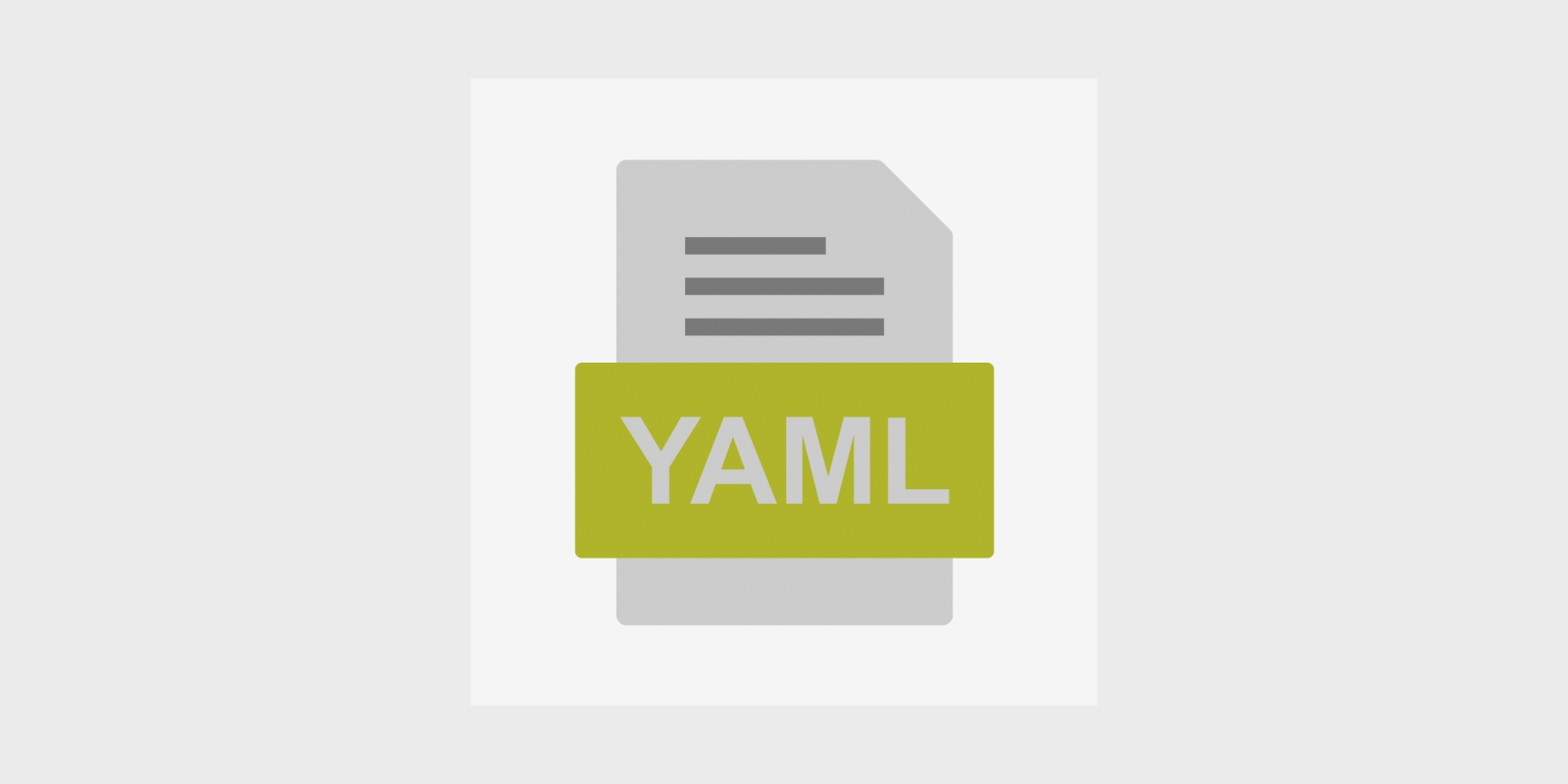
I’ll start by saying YAML is awesome! It’s like that thing you’ve been looking for but never knew existed, at least for my use case.
Url Protocol
Automatically Add a Protocol to a Url String in Angular
This little utility function can help you easily determine if a url string contains a protocol and add one if missing.
Waypoints
Using a directive to render Google Maps directions in Angular
A few months back while working on a location based taxi calling app, I had to build in functionality to render the route from the user’s location to the selected or searched listing on the map.
Window Object
How to get a Reference to the Window Object in an Angular 8 Application
There are many articles on the web showing various methods of getting a reference to the window object in Angular (primarily through the dependency injection mechanism). However those that are popular on Google search are from 2016, 2017, etc, and the methods are mostly overly complicated (understandably).
YAML
How YAML Helped Me Format and Process Text Data From Documents Much Faster
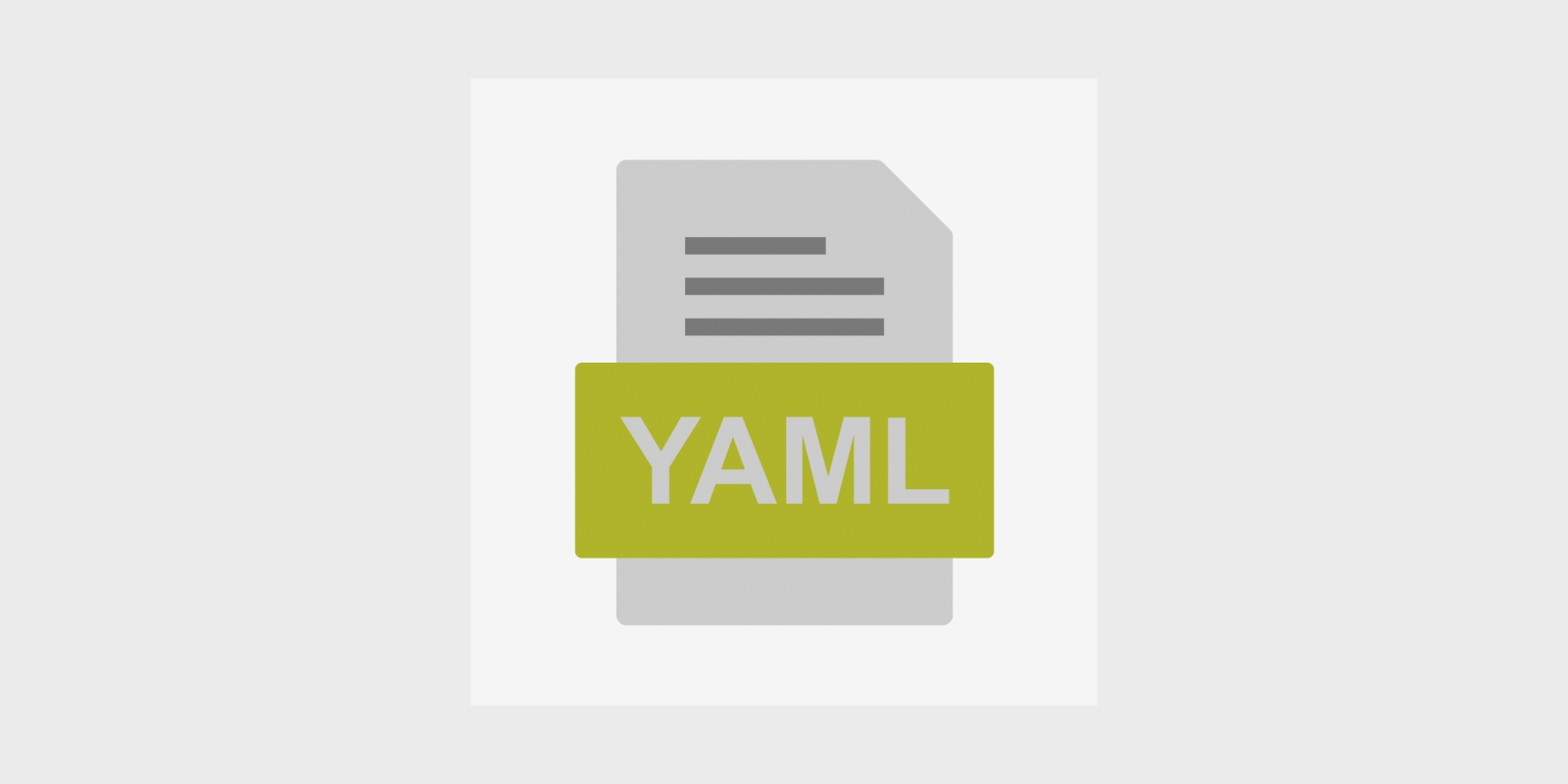
I’ll start by saying YAML is awesome! It’s like that thing you’ve been looking for but never knew existed, at least for my use case.
agGrid
Back to Top ↑agGrid Checkbox Selection
Back to Top ↑distinctUntilChanged
An in-depth look at RxJS’s distinctUntilChanged Operator
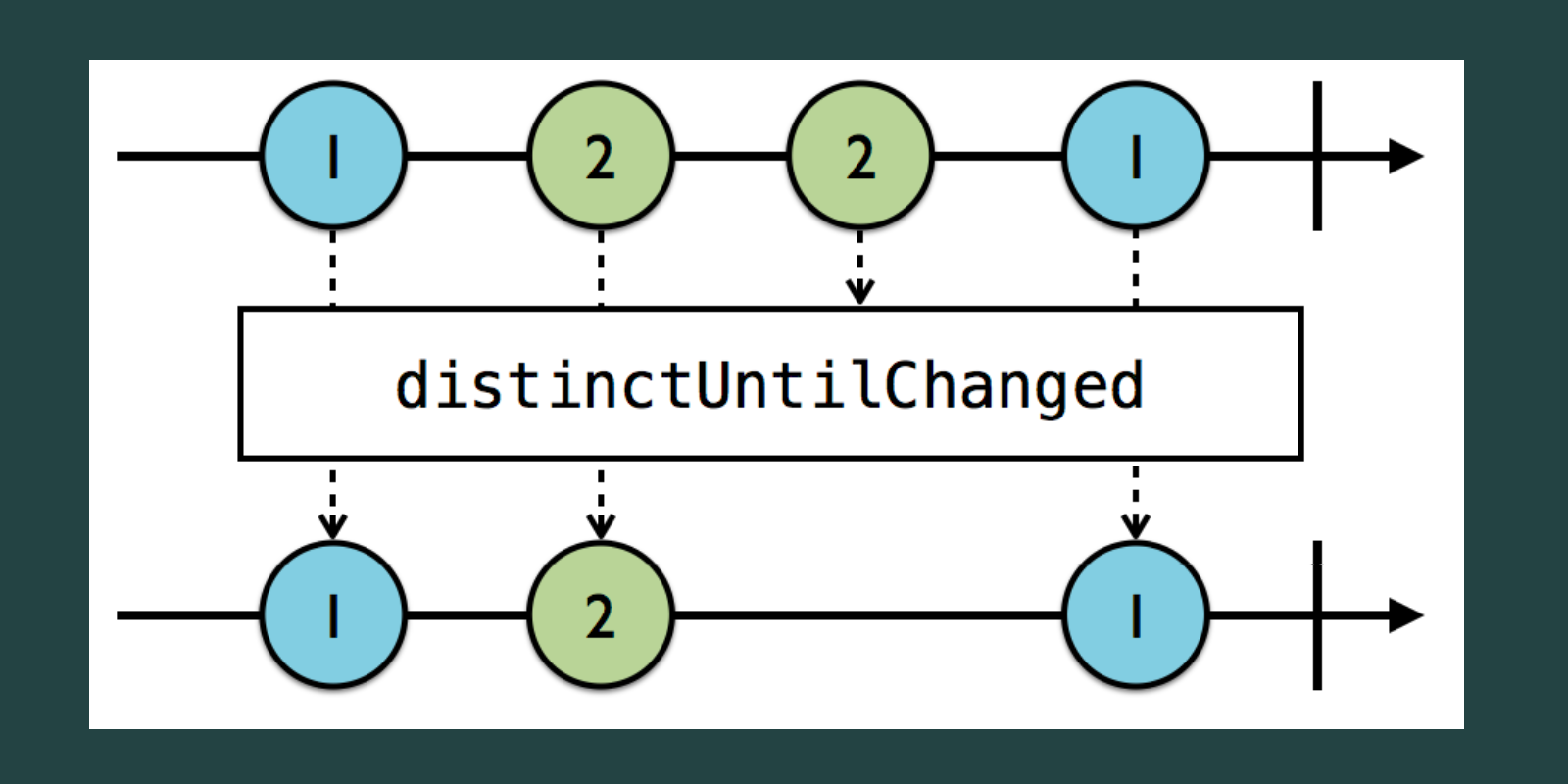
In this post, we’ll have an in-depth look at RxJS’s distinctUntilChanged
operator, it’s signature and what it does, it’s parameters compare
and keySelector
, and typical use cases for each of them and both of them.
html time
Using the html <time></time> element with Angular
<time [dateTime]="'2019-08-09 16:22:20'">8/9/2019</time>
jsPDF
Angular 2+ PDF Generation with jsPDF - Sample Project
I may flesh this out with a detailed post later, but if you have any questions or encounter any issues feel free to reach out to me.
regex
Detecting CAMTEL Numbers with Regex
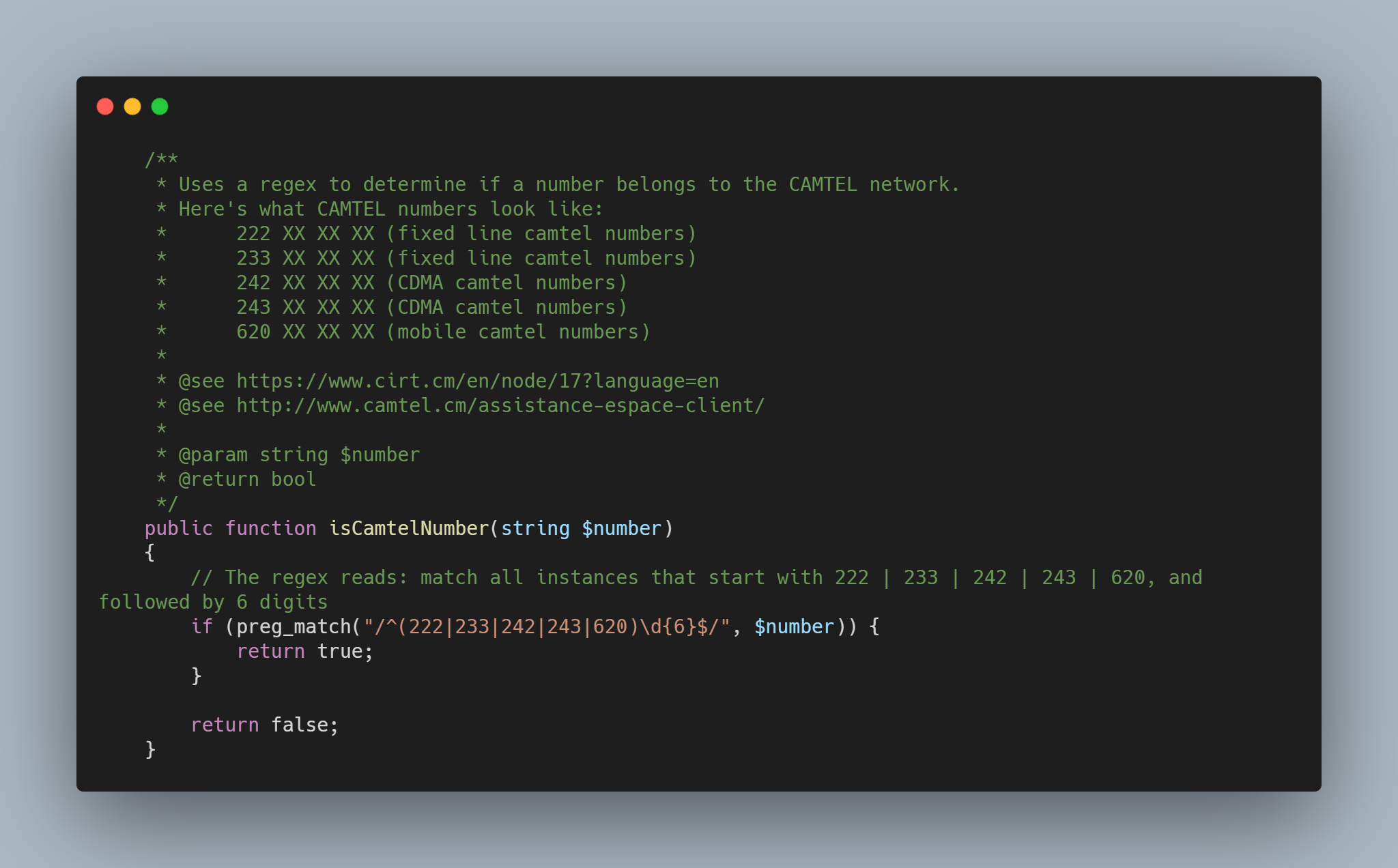
```php <?php
trackBy
Why you should use trackBy with Angular’s *ngFor loop
trackBy is a function which will return a unique identifier for each item in the array provided to *ngFor.
Normally when the array changes, Angular re-renders the whole DOM tree. But if you use trackBy, Angular will know which element has changed and will only make DOM changes for that particular element.
try/catch
On Exception Handling with try/catch in Javascript
Early today was researching why I don’t see a lot of exception handling with try/catch in Javascript codebases.